用c++写一个发送邮件到QQ邮箱的示例
时间: 2023-02-09 11:08:44 浏览: 95
你好!下面是一个使用 C 语言发送邮件到 QQ 邮箱的示例:
首先,你需要安装和配置 libcurl 库,这是一个用于处理网络传输的库,支持多种协议,包括 SMTP。你可以在这里获取 libcurl 库:https://curl.haxx.se/libcurl/
然后,你可以使用下面的代码来发送邮件:
```
#include <stdio.h>
#include <curl/curl.h>
int main(void)
{
CURL *curl;
CURLcode res;
curl = curl_easy_init();
if(curl) {
curl_easy_setopt(curl, CURLOPT_URL, "smtp://smtp.qq.com:587");
curl_easy_setopt(curl, CURLOPT_USE_SSL, (long)CURLUSESSL_ALL);
curl_easy_setopt(curl, CURLOPT_USERNAME, "your-email@qq.com");
curl_easy_setopt(curl, CURLOPT_PASSWORD, "your-email-password");
curl_easy_setopt(curl, CURLOPT_MAIL_FROM, "from@example.com");
struct curl_slist *recipients = NULL;
recipients = curl_slist_append(recipients, "to@example.com");
curl_easy_setopt(curl, CURLOPT_MAIL_RCPT, recipients);
curl_easy_setopt(curl, CURLOPT_READFUNCTION, NULL);
curl_easy_setopt(curl, CURLOPT_VERBOSE, 1L);
curl_easy_setopt(curl, CURLOPT_POSTFIELDS, "To: to@example.com\r\n"
"Subject: Test email using libcurl\r\n"
"\r\n"
"This is a test email message.\r\n");
res = curl_easy_perform(curl);
/* Check for errors */
if(res != CURLE_OK)
fprintf(stderr, "curl_easy_perform() failed: %s\n",
curl_easy_strerror(res));
/* Free the list of recipients */
curl_slist_free_all(recipients);
/* Always cleanup */
curl_easy_cleanup(curl);
}
return (int)res;
}
``
相关推荐
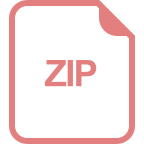
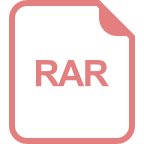
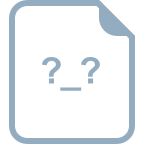
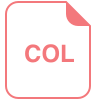
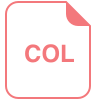
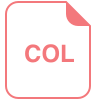
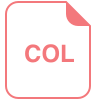
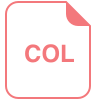









