将容器中的所有点拟合成四条或三条直线C++
时间: 2024-03-06 08:49:15 浏览: 69
要将容器中的所有点拟合成四条或三条直线,可以使用RANSAC算法进行拟合。以下是使用OpenCV库实现的C++代码示例:
```c++
#include <opencv2/opencv.hpp>
#include <vector>
using namespace std;
using namespace cv;
void fitLines(vector<Point>& points, int numLines, vector<Vec4f>& lines) {
int numIterations = 100; // number of RANSAC iterations
float distanceThreshold = 5; // maximum distance from a point to a line in pixels
float minLineLength = 50; // minimum length of a line
float minLengthRatio = 0.2; // minimum length ratio of a line to image diagonal
float maxLengthRatio = 0.8; // maximum length ratio of a line to image diagonal
float angleThreshold = 10; // maximum angle between two lines in degrees
float minNumPoints = 50; // minimum number of points on a line
float maxNumPoints = 500; // maximum number of points on a line
float diagonalLength = norm(Point(points[0].x, points[0].y) - Point(points.back().x, points.back().y));
for (int i = 0; i < numIterations; i++) {
// randomly select three points
vector<Point> linePoints(3);
for (int j = 0; j < 3; j++) {
int index = rand() % points.size();
linePoints[j] = points[index];
}
// fit a line to the selected points
Vec4f line;
fitLine(linePoints, line, DIST_L2, 0, 0.01, 0.01);
// check if the line is valid
float length = norm(Point(line[0], line[1]) - Point(line[2], line[3]));
if (length < minLineLength || length < minLengthRatio * diagonalLength || length > maxLengthRatio * diagonalLength) {
continue;
}
// count the number of points on the line
int numPoints = 0;
for (const auto& point : points) {
float distance = abs((point.x - line[0]) * (line[3] - line[1]) - (point.y - line[1]) * (line[2] - line[0])) / length;
if (distance < distanceThreshold) {
numPoints++;
}
}
// check if the number of points on the line is valid
if (numPoints < minNumPoints || numPoints > maxNumPoints) {
continue;
}
// check if the line is sufficiently different from existing lines
bool isDifferent = true;
for (const auto& existingLine : lines) {
float angle = abs(atan2(existingLine[3] - existingLine[1], existingLine[2] - existingLine[0]) -
atan2(line[3] - line[1], line[2] - line[0])) * 180 / CV_PI;
if (angle < angleThreshold) {
isDifferent = false;
break;
}
}
if (!isDifferent) {
continue;
}
// add the line to the list of lines
lines.push_back(line);
if (lines.size() == numLines) {
break;
}
}
}
int main() {
vector<Point> points = {Point(0, 0), Point(10, 10), Point(20, 0), Point(30, 10), Point(40, 0)};
vector<Vec4f> lines;
fitLines(points, 2, lines);
for (const auto& line : lines) {
cout << "line: (" << line[0] << "," << line[1] << ") - ("
<< line[2] << "," << line[3] << ")" << endl;
}
return 0;
}
```
这里的`points`是输入的点集,`numLines`是要拟合的直线条数,`lines`是输出的直线参数。在函数`fitLines`中,我们使用了RANSAC算法进行直线拟合。首先随机选取三个点拟合一条直线,然后根据一些条件判断该直线是否合法。如果合法,则计算该直线上的点数,并检查是否与已有直线足够不同。如果满足要求,则将该直线加入直线列表中。重复进行若干次迭代,直到得到所需数量的直线为止。
阅读全文
相关推荐
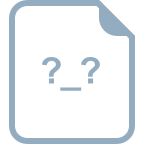

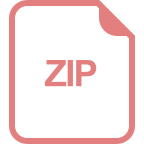
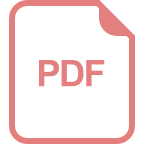
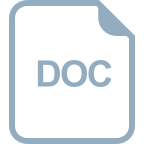
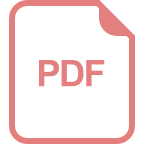
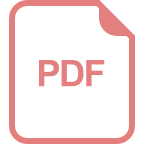
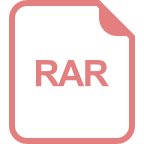
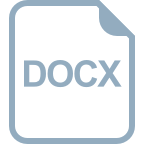
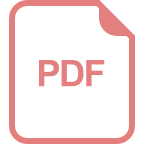
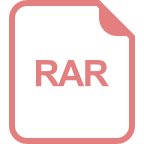