选择Swing
时间: 2023-07-02 22:15:21 浏览: 43
好的,下面我给您提供一个使用Swing编写的图形界面程序,实现姓名、学号、班级、性别、兴趣爱好的输入和展示:
```java
import javax.swing.*;
import java.awt.*;
public class StudentInfoGUI extends JFrame {
private JLabel nameLabel, idLabel, classLabel, genderLabel, hobbyLabel;
private JTextField nameField, idField;
private JComboBox<String> classComboBox;
private JRadioButton maleRadio, femaleRadio;
private JCheckBox sportBox, musicBox, artBox;
private JButton submitButton, clearButton;
public StudentInfoGUI() {
initUI();
setTitle("学生信息录入");
setSize(400, 300);
setLocationRelativeTo(null);
setDefaultCloseOperation(EXIT_ON_CLOSE);
setVisible(true);
}
private void initUI() {
//初始化组件
nameLabel = new JLabel("姓名:");
idLabel = new JLabel("学号:");
classLabel = new JLabel("班级:");
genderLabel = new JLabel("性别:");
hobbyLabel = new JLabel("兴趣爱好:");
nameField = new JTextField(10);
idField = new JTextField(10);
classComboBox = new JComboBox<>();
classComboBox.addItem("计算机科学与技术");
classComboBox.addItem("软件工程");
classComboBox.addItem("网络工程");
maleRadio = new JRadioButton("男");
femaleRadio = new JRadioButton("女");
ButtonGroup genderGroup = new ButtonGroup();
genderGroup.add(maleRadio);
genderGroup.add(femaleRadio);
sportBox = new JCheckBox("运动");
musicBox = new JCheckBox("音乐");
artBox = new JCheckBox("艺术");
submitButton = new JButton("提交");
clearButton = new JButton("清空");
//设置布局
setLayout(new GridBagLayout());
GridBagConstraints c = new GridBagConstraints();
c.gridx = 0;
c.gridy = 0;
c.insets = new Insets(10, 10, 10, 10);
add(nameLabel, c);
c.gridx = 1;
add(nameField, c);
c.gridx = 0;
c.gridy = 1;
add(idLabel, c);
c.gridx = 1;
add(idField, c);
c.gridx = 0;
c.gridy = 2;
add(classLabel, c);
c.gridx = 1;
add(classComboBox, c);
c.gridx = 0;
c.gridy = 3;
add(genderLabel, c);
c.gridx = 1;
JPanel genderPanel = new JPanel();
genderPanel.add(maleRadio);
genderPanel.add(femaleRadio);
add(genderPanel, c);
c.gridx = 0;
c.gridy = 4;
add(hobbyLabel, c);
c.gridx = 1;
JPanel hobbyPanel = new JPanel();
hobbyPanel.setLayout(new GridLayout(3, 1));
hobbyPanel.add(sportBox);
hobbyPanel.add(musicBox);
hobbyPanel.add(artBox);
add(hobbyPanel, c);
c.gridx = 0;
c.gridy = 5;
add(submitButton, c);
c.gridx = 1;
add(clearButton, c);
}
public static void main(String[] args) {
new StudentInfoGUI();
}
}
```
这段代码中,我们使用了GridLayout和GridBagConstraints来设置图形界面的布局。其中,姓名、学号、班级、性别、兴趣爱好的输入框和下拉菜单都是使用了Swing提供的组件实现的。
在这个程序中,我们通过JRadioButton和ButtonGroup来实现了性别的单选功能,通过JCheckBox来实现了兴趣爱好的多选功能。提交和清空按钮则是使用了JButton组件。
这个程序的界面比较简单,您可以根据需要自行修改样式和布局。对于数据的存储,您可以使用文件或数据库等方式进行存储。
相关推荐
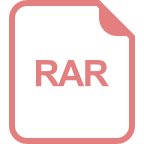














