在.net standerd 怎么使用remoting
时间: 2024-03-06 20:50:26 浏览: 16
在 .NET Standard 中使用 Remoting 需要使用 System.Runtime.Remoting 命名空间下的类。以下是使用 Remoting 的基本步骤:
1. 创建一个 Remoting 服务端应用程序,并在其中定义一个远程对象。使用 System.Runtime.Remoting.RemotingConfiguration.Configure() 方法来启用 Remoting 支持。
2. 在客户端应用程序中,使用 System.Runtime.Remoting.RemotingConfiguration.RegisterWellKnownClientType() 方法来注册远程对象的类型,并使用 Activator.GetObject() 方法来获取远程对象的实例。
3. 通过远程对象的代理,客户端可以调用远程对象的方法。
以下是一个简单的示例代码:
服务端应用程序:
```csharp
using System;
using System.Runtime.Remoting;
using System.Runtime.Remoting.Channels;
using System.Runtime.Remoting.Channels.Tcp;
namespace RemotingServer
{
class Program
{
static void Main(string[] args)
{
TcpServerChannel channel = new TcpServerChannel(8080);
ChannelServices.RegisterChannel(channel, false);
RemotingConfiguration.RegisterWellKnownServiceType(
typeof(MyService), "MyService", WellKnownObjectMode.Singleton);
Console.WriteLine("Remoting server is running...");
Console.ReadLine();
}
}
public class MyService : MarshalByRefObject
{
public string SayHello(string name)
{
return $"Hello, {name}!";
}
}
}
```
客户端应用程序:
```csharp
using System;
using System.Runtime.Remoting;
namespace RemotingClient
{
class Program
{
static void Main(string[] args)
{
RemotingConfiguration.RegisterWellKnownClientType(
typeof(MyService), "tcp://localhost:8080/MyService");
MyService service = (MyService)Activator.GetObject(
typeof(MyService), "tcp://localhost:8080/MyService");
string result = service.SayHello("World");
Console.WriteLine(result);
Console.ReadLine();
}
}
public class MyService : MarshalByRefObject
{
public string SayHello(string name)
{
return $"Hello, {name}!";
}
}
}
```
在上面的示例中,服务端应用程序创建了一个名为 MyService 的远程对象,并将其注册为单例模式。客户端应用程序使用 RemotingConfiguration.RegisterWellKnownClientType() 方法注册远程对象类型,并使用 Activator.GetObject() 方法获取远程对象的实例。然后,客户端应用程序通过远程对象的代理调用 MyService.SayHello() 方法,该方法返回一个字符串,表示问候语。
相关推荐
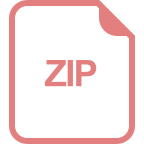
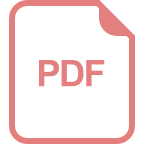

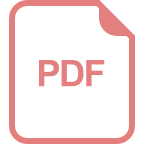
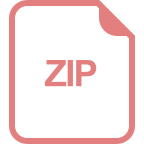
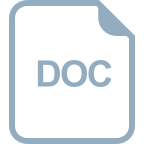
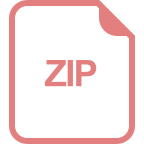
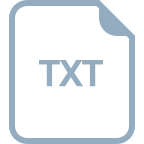
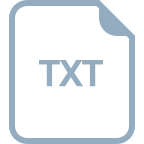
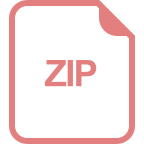
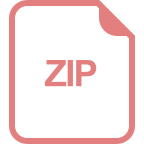
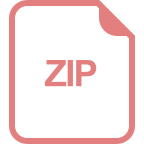