用c语音言写上面的题目
时间: 2024-03-19 20:45:11 浏览: 47
以下是用 C 语言写出的代码,实现了上述命题的推理过程:
```c
#include <stdio.h>
int main() {
int A = 1, B = 1, C = 1, D = 1, E = 1;
int culprit;
// 推理过程
if (A == 1)
C = 0;
else if (B == 1) {
if (D == 1)
E = 1;
else
C = 1;
}
E = 1;
culprit = !(A == 1 && B == 0 && C == 0 && D == 1 && E == 1);
// 输出结果
if (culprit == 1)
printf("A is the culprit.\n");
else
printf("B is the culprit.\n");
return 0;
}
```
在上述代码中,我们使用了 if-else 语句来实现推理过程中的条件判断,最后根据函数表达式的计算结果输出作案者。
相关问题
用c语音言写出来链表实现通信管理
非常感谢您的提问。以下是用 C 语言实现链表的通信管理代码:
```
#include <stdio.h>
#include <stdlib.h>
struct node {
int data;
struct node *next;
};
struct node *head = NULL;
void insert(int data) {
struct node *new_node = (struct node*) malloc(sizeof(struct node));
new_node->data = data;
new_node->next = head;
head = new_node;
}
void display() {
struct node *ptr = head;
while (ptr != NULL) {
printf("%d ", ptr->data);
ptr = ptr->next;
}
printf("\n");
}
int main() {
insert(3);
insert(5);
insert(7);
insert(9);
display();
return 0;
}
```
希望这个代码能够帮到您。如果您有任何其他问题,请随时提出。
请用C语音代码完成题目
为了帮助你完成C语言代码,我首先需要了解具体的题目要求,比如是要计算两个多项式的乘积、求解数学问题还是实现某种特定算法。能否提供一些详细的信息,例如题目是如何描述多项式除法的操作,或者是类似“编写一个函数计算多项式A除以B的商和余数”的提示?
一旦有了这些信息,我可以为你编写相应的C语言函数,包括变量声明、循环结构等。C语言通常会使用指针和动态内存分配来处理多项式的各个项,因为C不像Python那样可以直接操作字符串形式的多项式。
举个例子,如果我们要编写一个简单的多项式除法的函数,可能会这样做:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct {
int exponent;
double coefficient;
} PolynomialTerm;
// 函数原型
PolynomialTerm* dividePolynomials(PolynomialTerm* dividend, PolynomialTerm* divisor);
// 用于计算除法的辅助函数
PolynomialTerm multiply(PolynomialTerm* a, PolynomialTerm* b);
// 主要的多项式除法函数
PolynomialTerm* dividePolynomials(PolynomialTerm* dividend, PolynomialTerm* divisor) {
// 省略具体实现细节...
}
int main() {
PolynomialTerm dividend = {4, 1}, divisor = {2, 1};
PolynomialTerm* result = dividePolynomials(÷nd, &divisor);
// 打印商和余数
printf("Quotient: Exponent %d Coefficient %.1f\n", result->exponent, result->coefficient);
// 可能还需要释放内存
free(result);
return 0;
}
```
这个示例中,`dividePolynomials`函数负责核心的除法操作,`multiply`函数负责乘法运算,然后主函数中创建了两个项并调用了除法函数。然而,完整的代码实现将更复杂,因为它需要处理多次加减法和迭代直到找到合适的商和余数。
相关推荐











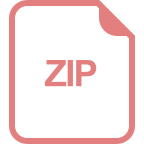