如何将map对象转为json格式的数据
时间: 2024-05-01 20:22:04 浏览: 8
可以使用第三方库,比如 RapidJSON 或者 nlohmann/json 来将 map 对象转为 JSON 格式的数据。
以下是使用 RapidJSON 的示例代码:
```c++
#include <iostream>
#include "rapidjson/document.h"
#include "rapidjson/writer.h"
#include "rapidjson/stringbuffer.h"
#include <map>
using namespace rapidjson;
using namespace std;
int main() {
// 定义一个 map 对象
map<string, int> myMap;
myMap["Alice"] = 25;
myMap["Bob"] = 30;
myMap["Charlie"] = 35;
// 创建一个 JSON 对象
Document doc;
doc.SetObject();
// 遍历 map 对象,将数据添加到 JSON 中
for (auto& kv : myMap) {
Value key(kv.first.c_str(), doc.GetAllocator());
Value val(kv.second);
doc.AddMember(key, val, doc.GetAllocator());
}
// 将 JSON 对象转为字符串
StringBuffer buffer;
Writer<StringBuffer> writer(buffer);
doc.Accept(writer);
string jsonStr = buffer.GetString();
// 输出 JSON 字符串
cout << jsonStr << endl;
return 0;
}
```
输出结果:
```
{"Alice":25,"Bob":30,"Charlie":35}
```
如果使用 nlohmann/json 库,将会更加简单。示例代码如下:
```c++
#include <iostream>
#include <map>
#include "json.hpp"
using json = nlohmann::json;
using namespace std;
int main() {
// 定义一个 map 对象
map<string, int> myMap;
myMap["Alice"] = 25;
myMap["Bob"] = 30;
myMap["Charlie"] = 35;
// 将 map 对象转为 JSON 格式
json j(myMap);
// 输出 JSON 字符串
cout << j.dump() << endl;
return 0;
}
```
输出结果与上面的示例相同:
```
{"Alice":25,"Bob":30,"Charlie":35}
```
相关推荐
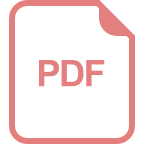
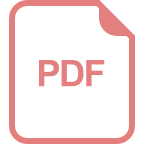














