c++加法运算符重载电子钟表
时间: 2023-10-18 12:07:52 浏览: 108
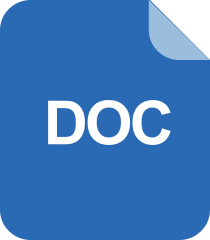
c++运算符重载
好的,我可以为你提供一些代码示例来演示如何在 C++ 中重载加法运算符来实现电子钟表。
首先,我们需要定义一个 `Time` 类来表示时间,包括小时、分钟和秒:
```cpp
class Time {
public:
Time(int h = 0, int m = 0, int s = 0)
: hour(h), minute(m), second(s) {}
int hour;
int minute;
int second;
};
```
然后,我们可以重载 `operator+` 运算符,使得两个 `Time` 对象相加后返回一个新的 `Time` 对象。实现方式如下:
```cpp
Time operator+ (const Time& t1, const Time& t2) {
int total_seconds = t1.hour * 3600 + t1.minute * 60 + t1.second
+ t2.hour * 3600 + t2.minute * 60 + t2.second;
int h = total_seconds / 3600;
int m = (total_seconds % 3600) / 60;
int s = total_seconds % 60;
return Time(h, m, s);
}
```
上述代码中,我们将两个 `Time` 对象的小时、分钟和秒分别相加,然后将总秒数转换为新的 `Time` 对象并返回。
最后,我们可以编写一个简单的测试程序来测试我们的代码:
```cpp
int main() {
Time t1(10, 30, 0);
Time t2(0, 45, 30);
Time t3 = t1 + t2;
cout << "t1: " << t1.hour << ":" << t1.minute << ":" << t1.second << endl;
cout << "t2: " << t2.hour << ":" << t2.minute << ":" << t2.second << endl;
cout << "t1 + t2 = " << t3.hour << ":" << t3.minute << ":" << t3.second << endl;
return 0;
}
```
运行上述代码,输出结果如下:
```
t1: 10:30:0
t2: 0:45:30
t1 + t2 = 11:15:30
```
在这个示例中,我们演示了如何使用 C++ 中的运算符重载来实现电子钟表的加法运算。
阅读全文
相关推荐
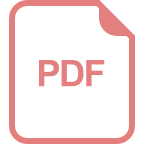
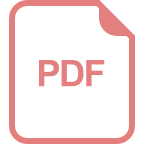
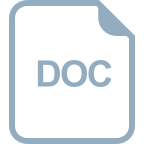
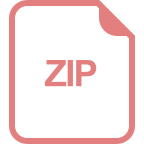
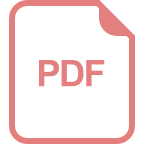
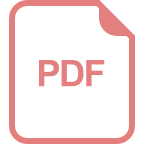
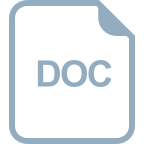
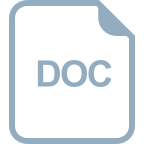