java 树形结构统计
时间: 2023-10-14 14:10:28 浏览: 40
Java中可以使用递归来实现树形结构的统计。假设我们的树结构如下:
```
- A
- B
- D
- E
- C
- F
```
可以定义一个节点类来表示每个节点:
```java
class Node {
String value;
List<Node> children;
public Node(String value) {
this.value = value;
children = new ArrayList<>();
}
public void addChild(Node child) {
children.add(child);
}
}
```
然后我们可以使用递归来统计树的节点数。统计节点数的方法可以定义在节点类中,也可以定义在外部类中。
```java
public int countNodes(Node node) {
if (node == null) {
return 0;
}
int count = 1; // 当前节点也算一个
for (Node child : node.children) {
count += countNodes(child);
}
return count;
}
```
在上面的例子中,调用`countNodes(A)`会返回6,因为树中有6个节点。
我们也可以使用类似的递归方式来统计树的深度(即最大层数):
```java
public int maxDepth(Node node) {
if (node == null) {
return 0;
}
int maxChildDepth = 0;
for (Node child : node.children) {
int childDepth = maxDepth(child);
if (childDepth > maxChildDepth) {
maxChildDepth = childDepth;
}
}
return maxChildDepth + 1; // 当前节点也算一层
}
```
在上面的例子中,调用`maxDepth(A)`会返回3,因为树的最大深度为3。
相关推荐
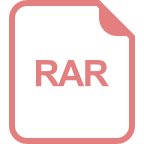
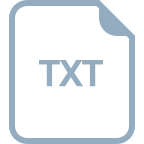
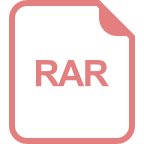














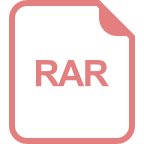