用Python实现文献Tania, S., Murshed, M., Teng, S. W., & Karmakar, G. (2020). An Enhanced Local Texture Descriptor for Image Segmentation. 2020 IEEE International Conference on Image Processing (ICIP). doi:10.1109/icip40778.2020.9190895的算法
时间: 2023-12-06 19:42:24 浏览: 34
这篇文章提出了一种用于图像分割的增强局部纹理描述符(Enhanced Local Texture Descriptor,简称ELTD)。下面是基于Python实现的ELTD算法的代码:
```python
import cv2
import numpy as np
def eltd(image, window_size=3, num_bins=8, sigma=0.2):
# 将图像转换成灰度图
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# 计算图像的梯度
gx = cv2.Sobel(gray, cv2.CV_64F, 1, 0, ksize=3)
gy = cv2.Sobel(gray, cv2.CV_64F, 0, 1, ksize=3)
# 计算像素点的梯度幅值和方向
mag, ang = cv2.cartToPolar(gx, gy, angleInDegrees=True)
# 将角度调整到0-180度之间
ang = np.mod(ang, 180)
# 计算每个像素点的ELTD特征向量
height, width = gray.shape
eltd_features = np.zeros((height, width, num_bins))
half_window = window_size // 2
for i in range(half_window, height - half_window):
for j in range(half_window, width - half_window):
# 计算当前窗口内的梯度幅值和方向
mag_window = mag[i-half_window:i+half_window+1, j-half_window:j+half_window+1]
ang_window = ang[i-half_window:i+half_window+1, j-half_window:j+half_window+1]
# 将方向值划分到不同的bin中
bins = np.floor(ang_window / (180 / num_bins)).astype(int)
# 计算当前像素点的ELTD特征向量
for k in range(num_bins):
eltd_features[i, j, k] = np.sum(mag_window[bins == k])
# 对特征向量进行归一化
eltd_features /= np.linalg.norm(eltd_features, axis=2, keepdims=True) + 1e-6
# 对特征向量进行平滑处理
eltd_features = cv2.GaussianBlur(eltd_features, (0, 0), sigma)
# 返回ELTD特征
return eltd_features
```
这段代码实现了ELTD算法的主要逻辑,它接受一张彩色图像作为输入,然后返回ELTD特征。其中,`window_size`参数表示局部纹理窗口的大小,`num_bins`参数表示将方向值划分到多少个bin中,`sigma`参数表示平滑处理的高斯核标准差。
需要注意的是,ELTD算法的实现还需要结合其他图像分割算法一起使用,才能得到最终的分割结果。例如,可以使用基于聚类的方法对ELTD特征进行聚类,得到分割结果。
相关推荐
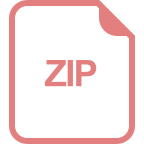
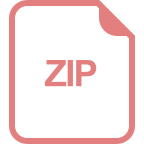
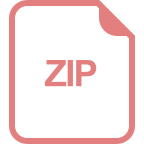
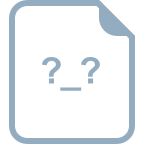
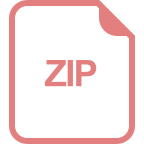
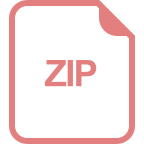
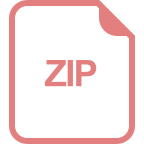
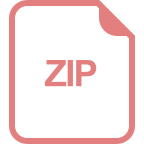
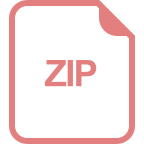
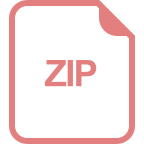