请完成下面题目并给出思路及代码:用C++设计一个地图,不要用面向对象,地图中包含障碍物、怪物和宝藏等元素,可以使用特定的字符来代表不同元素,地图大小不小于10 x 10。 玩家可以通过输入 W、A、S、D 来分别向上、向左、向下、向右移动。在地图上增加障碍物和怪物等阻挡玩家前进的障碍,其中障碍物表示玩家无法通过,可以用“#”来表示,玩家如果碰到怪物游戏就会结束,怪物可以采用“@”符号来表示。玩家需要躲避这些障碍和怪物,并到达宝藏的位置,以完成游戏。 要求:1.如果移动的位置上有障碍物,则玩家不能通过。 2. 玩家遇到怪物时,需要躲避,如果不小心撞上了,游戏结束。 3. 玩家到达宝藏的位置即可完成游戏。 4. 玩家可以通过指令保存游戏进度,下次进入游戏时可以继续游戏。 5.采用函数对整个游戏进行解构,用C++实现,不要用面向对象,多利用函数,地图的大小用动态输入二维数组。
时间: 2024-03-24 20:40:19 浏览: 79
思路:
1. 定义常量表示地图的大小,使用动态输入二维数组来存储地图,使用随机数生成障碍、怪物和宝藏的位置,使用特定字符表示不同元素。
2. 定义一个玩家结构体,包含玩家当前位置和生命值等信息。
3. 定义函数来实现玩家移动、碰撞检测等功能。
4. 定义函数来实现游戏的保存和读取功能。
5. 在主函数中调用函数实现游戏流程。
代码实现:
```c++
#include <iostream>
#include <ctime>
#include <cstdlib>
using namespace std;
const int MAP_SIZE = 10;
const char OBSTACLE = '#';
const char MONSTER = '@';
const char TREASURE = '$';
struct Player {
int x, y;
int hp;
};
// 初始化地图
void initMap(char map[][MAP_SIZE]) {
srand((unsigned)time(NULL));
for (int i = 0; i < MAP_SIZE; i++) {
for (int j = 0; j < MAP_SIZE; j++) {
map[i][j] = '.';
}
}
for (int i = 0; i < MAP_SIZE; i++) {
int x = rand() % MAP_SIZE;
int y = rand() % MAP_SIZE;
if (map[x][y] != '.') {
i--;
continue;
}
map[x][y] = OBSTACLE;
}
for (int i = 0; i < MAP_SIZE; i++) {
int x = rand() % MAP_SIZE;
int y = rand() % MAP_SIZE;
if (map[x][y] != '.') {
i--;
continue;
}
map[x][y] = MONSTER;
}
for (int i = 0; i < MAP_SIZE; i++) {
int x = rand() % MAP_SIZE;
int y = rand() % MAP_SIZE;
if (map[x][y] != '.') {
i--;
continue;
}
map[x][y] = TREASURE;
}
}
// 显示地图
void showMap(char map[][MAP_SIZE]) {
for (int i = 0; i < MAP_SIZE; i++) {
for (int j = 0; j < MAP_SIZE; j++) {
cout << map[i][j] << ' ';
}
cout << endl;
}
}
// 移动玩家
void movePlayer(Player& player, char map[][MAP_SIZE], char direction) {
int x = player.x, y = player.y;
switch (direction) {
case 'W':
case 'w':
x--;
break;
case 'A':
case 'a':
y--;
break;
case 'S':
case 's':
x++;
break;
case 'D':
case 'd':
y++;
break;
default:
break;
}
if (x < 0 || x >= MAP_SIZE || y < 0 || y >= MAP_SIZE) {
cout << "越界了!" << endl;
return;
}
if (map[x][y] == OBSTACLE) {
cout << "遇到障碍物了!" << endl;
return;
}
if (map[x][y] == MONSTER) {
cout << "遇到怪物了!" << endl;
player.hp--;
if (player.hp <= 0) {
cout << "你死了!" << endl;
exit(0);
}
return;
}
if (map[x][y] == TREASURE) {
cout << "你找到了宝藏!" << endl;
exit(0);
}
player.x = x;
player.y = y;
}
// 保存游戏进度
void saveGame(Player player) {
FILE* file = fopen("savedata.txt", "wb");
fwrite(&player, sizeof(Player), 1, file);
fclose(file);
cout << "保存成功!" << endl;
}
// 读取游戏进度
Player loadGame() {
Player player;
FILE* file = fopen("savedata.txt", "rb");
if (file == NULL) {
cout << "没有保存的游戏数据!" << endl;
player.hp = 3;
player.x = 0;
player.y = 0;
return player;
}
fread(&player, sizeof(Player), 1, file);
fclose(file);
cout << "读取成功!" << endl;
return player;
}
int main() {
char map[MAP_SIZE][MAP_SIZE];
initMap(map);
showMap(map);
Player player = loadGame();
while (true) {
char direction;
cout << "请输入移动方向(WASD):";
cin >> direction;
movePlayer(player, map, direction);
showMap(map);
saveGame(player);
}
return 0;
}
```
阅读全文
相关推荐
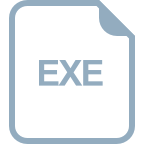
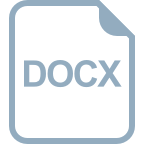
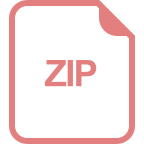
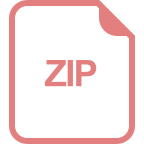
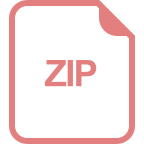
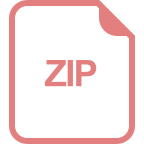
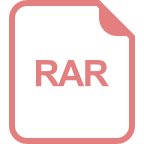
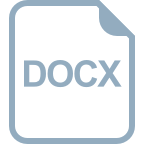
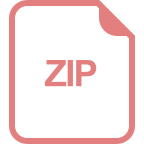
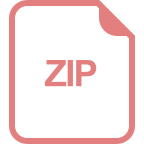
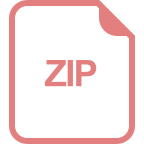
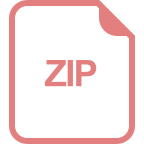
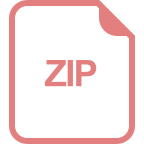
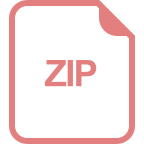
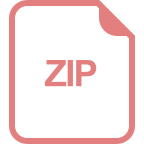