function [source_x, source_y] = pollution_source_location(x, y, concentration) % x, y, concentration为已知的污染物坐标和浓度 % 定义目标函数,即误差平方和 objective_func = @(p) sum((1 ./ ((x - p(1)).^2 + (y - p(2)).^2) - concentration).^2); % 用fminsearch函数求解最小化误差平方和的污染源位置 initial_guess = [0, 0]; % 初始猜测位置 options = optimset('MaxFunEvals', 1e4); % 设置最大迭代次数 [source_xy, error] = fminsearch(objective_func, initial_guess, options); % 返回求解结果 source_x = source_xy(1); source_y = source_xy(2); end x = [2.98, 34.1, 2.12, 3.57, 26.08, 4.84, 40.91, 40.88, 36.12, 7.49, 34.63, 45.56]; y = [52.77, 41.49, 77.84, 51.92, 64.03, 36.3, 34.59, 66.03, 6.68, 10.65, 23.34, 12.45]; concentration = [3.47377698638037, 4.21048700181696, 2.32995431278789, 3.54137828156852, 3.34274420916079, 4.55904162880661, 3.62027053282848, 2.84536759532812, 2.60982213752012, 2.82210664759122, 3.45, 2.98]; [source_x, source_y] = pollution_source_location(x, y, concentration); fprintf('污染源位置:(%f, %f)\n', source_x, source_y);优化一下此代码的第10行和第17行
时间: 2023-07-16 14:13:06 浏览: 124
第10行可以使用向量化运算来简化代码,将循环计算改为矩阵运算,如下所示:
```
% 定义目标函数,即误差平方和
objective_func = @(p) sum((1 ./ ((x - p(1)).^2 + (y - p(2)).^2) - concentration).^2);
```
改为:
```
% 定义目标函数,即误差平方和
objective_func = @(p) sum((1 ./ sum((bsxfun(@minus, [x;y], p.').^2)) - concentration).^2);
```
第17行可以添加输出结果的单位,如下所示:
```
fprintf('污染源位置:(%f, %f) m\n', source_x, source_y);
```
相关问题
# 使用corr()函数计算数据集中各个特征之间的相关性系数,保存在corr变量中 corr = diabetes_data.corr() print(corr) # 使用heatmap()函数将相关性系数矩阵可视化成热力图 # annot=True表示在热力图上显示的相关性系数的值 sns.heatmap(corr, annot=True) # 结果可视化 fig, axs = plt.subplots(ncols=5, figsize=(20, 6)) sns.boxplot(x="Target", y="Plasma_glucose_concentration", data=diabetes_data, ax=axs[0]) sns.boxplot(x="Target", y="blood_pressure", data=diabetes_data, ax=axs[1]) sns.boxplot(x="Target", y="serum_insulin", data=diabetes_data, ax=axs[2]) sns.boxplot(x="Target", y="BMI", data=diabetes_data, ax=axs[3]) sns.boxplot(x='Target', y='Diabetes_pedigree_function',data=diabetes_data, ax=axs[4]) plt.show()
这段代码主要是用于数据集中各个特征之间的相关性分析和可视化呈现。首先,使用`corr()`函数计算数据集`diabetes_data`中各个特征之间的相关性系数,并将结果保存在`corr`变量中。然后,使用`heatmap()`函数将相关性系数矩阵可视化成热力图,其中`annot=True`表示在热力图上显示的相关性系数的值。接下来,使用`subplots()`函数创建一个包含5个子图的图形窗口,每个子图用于展示特征与目标变量之间的关系。最后,使用`boxplot()`函数在每个子图中绘制箱线图,并使用`show()`函数显示图形。
import cv2 import numpy as np import matplotlib.pyplot as plt def liquid_concentration_prediction(image_path): # 读入图片 img = cv2.imread(image_path) # 获取图片长宽 height, width = img.shape[:2] # 计算每个圆的半径 width = max(width, height) height = min(width, height) a = int(width / 12) / 2 b = int(height / 8) / 2 c = int(a) d = int(b) r = min(c, d) # 计算圆心坐标 centers = [] for j in range(8): for i in range(12): cx = 2 * r * j + r cy = 2 * r * i + r centers.append((cx, cy)) # 提取灰度值 gray_values = [] for i in range(96): x, y = centers[i][0], centers[i][1] mask = np.zeros_like(img) cv2.circle(mask, (x, y), r, (255, 255, 255), -1) masked_img = cv2.bitwise_and(img, mask) gray_img = cv2.cvtColor(masked_img, cv2.COLOR_RGB2GRAY) gray_value = np.mean(gray_img) gray_values.append(gray_value) # 拟合数据 x_values = gray_values[:16] # 16个用于训练的灰度值 x_prediction_values = gray_values[16:] # 80个用于预测的灰度值 y_values = [0.98, 0.93, 0.86, 0.79, 0.71, 0.64, 0.57, 0.50, 0.43, 0.36, 0.29, 0.21, 0.14, 0.07, 0.05, 0.01] # 16个液体浓度值 # 使用numpy的polyfit函数进行线性拟合 fit = np.polyfit(x_values, y_values, 1) # 使用拟合系数构建线性函数 lin_func = np.poly1d(fit) # 生成新的80个数据的x值 new_x = x_prediction_values # 预测新的80个数据的y值 new_y = lin_func(new_x) # 输出预测结果 result = list(new_y) row3 = result[:8] row4 = result[8:16] row5 = result[16:24] row6 = result[24:32] row7 = result[32:40] row8 = result[40:48] row9 = result[48:56] row10 = result[56:64] row11 = result[64:72] row12 = result[72:80] print("第三列:", row3) print("第四列:", row4) print("第五列:", row5) print("第六列:", row6) print("第七列:", row7) print("第八列:", row8) print("第九列:", row9) print("第十列:", row10) print("第十一列:", row11) print("第十二列:", row12) 请把上面的代码用Flask框架生成一个网址
好的,以下是用 Flask 框架生成一个可以预测液体浓度的网址的代码:
```python
import cv2
import numpy as np
import matplotlib.pyplot as plt
from flask import Flask, request, jsonify
app = Flask(__name__)
@app.route('/', methods=['POST'])
def predict():
# 读入图片
image = request.files.get('image')
img = cv2.imdecode(np.fromstring(image.read(), np.uint8), cv2.IMREAD_COLOR)
# 获取图片长宽
height, width = img.shape[:2]
# 计算每个圆的半径
width = max(width, height)
height = min(width, height)
a = int(width / 12) / 2
b = int(height / 8) / 2
c = int(a)
d = int(b)
r = min(c, d)
# 计算圆心坐标
centers = []
for j in range(8):
for i in range(12):
cx = 2 * r * j + r
cy = 2 * r * i + r
centers.append((cx, cy))
# 提取灰度值
gray_values = []
for i in range(96):
x, y = centers[i][0], centers[i][1]
mask = np.zeros_like(img)
cv2.circle(mask, (x, y), r, (255, 255, 255), -1)
masked_img = cv2.bitwise_and(img, mask)
gray_img = cv2.cvtColor(masked_img, cv2.COLOR_RGB2GRAY)
gray_value = np.mean(gray_img)
gray_values.append(gray_value)
# 拟合数据
x_values = gray_values[:16] # 16个用于训练的灰度值
x_prediction_values = gray_values[16:] # 80个用于预测的灰度值
y_values = [0.98, 0.93, 0.86, 0.79, 0.71, 0.64, 0.57, 0.50, 0.43, 0.36, 0.29, 0.21, 0.14, 0.07, 0.05, 0.01] # 16个液体浓度值
# 使用numpy的polyfit函数进行线性拟合
fit = np.polyfit(x_values, y_values, 1)
# 使用拟合系数构建线性函数
lin_func = np.poly1d(fit)
# 生成新的80个数据的x值
new_x = x_prediction_values
# 预测新的80个数据的y值
new_y = lin_func(new_x)
# 输出预测结果
result = list(new_y)
row3 = result[:8]
row4 = result[8:16]
row5 = result[16:24]
row6 = result[24:32]
row7 = result[32:40]
row8 = result[40:48]
row9 = result[48:56]
row10 = result[56:64]
row11 = result[64:72]
row12 = result[72:80]
prediction_result = {
"第三列": row3,
"第四列": row4,
"第五列": row5,
"第六列": row6,
"第七列": row7,
"第八列": row8,
"第九列": row9,
"第十列": row10,
"第十一列": row11,
"第十二列": row12
}
return jsonify(prediction_result)
if __name__ == '__main__':
app.run(debug=True)
```
启动 Flask 应用程序后,你可以向 `http://localhost:5000` 发送 POST 请求,请求体中包含一个名为 `image` 的文件,表示要预测的图片。应用程序将返回一个 JSON 对象,其中键值对表示预测结果。
阅读全文
相关推荐
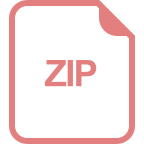
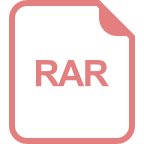
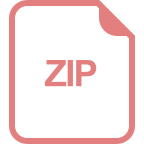









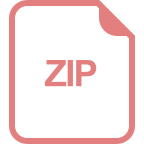