用bootstrap 和jq 写一个梯形的盒子,底部为反圆角
时间: 2024-03-21 20:40:34 浏览: 132
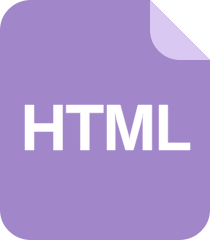
盒子模型代码
可以使用 Bootstrap 和 jQuery 来实现一个梯形的盒子,底部为反圆角,具体步骤如下:
1. 创建 HTML 结构,包含一个 div 元素作为容器。
```html
<div class="trapezoid"></div>
```
2. 添加 CSS 样式,设置容器的宽度、高度、边框和位置,并使用 transform 和 skewX 属性将元素变成一个梯形。
```css
.trapezoid {
width: 200px;
height: 100px;
border-top: 50px solid #ccc;
border-left: 25px solid transparent;
border-right: 25px solid transparent;
position: fixed;
bottom: 0;
left: 50%;
transform: translateX(-50%) skewX(-15deg);
}
```
3. 使用 jQuery 将容器的底部两个角设置为直角,并且添加一个点击事件,点击容器时切换底部两个角的样式。
```javascript
$(document).ready(function() {
$('.trapezoid').click(function() {
$(this).toggleClass('rounded-bottom');
});
});
```
4. 添加反圆角的 CSS 样式,将容器的底部左右两个角设置为直角,中间两个角设置为圆角。
```css
.rounded-bottom-left {
border-bottom-left-radius: 0 !important;
}
.rounded-bottom-right {
border-bottom-right-radius: 0 !important;
}
.rounded-bottom {
border-radius: 0 0 10px 10px !important;
}
```
完整代码如下:
```html
<!DOCTYPE html>
<html>
<head>
<title>Bootstrap Trapezoid</title>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<link rel="stylesheet" href="https://cdn.staticfile.org/twitter-bootstrap/4.5.3/css/bootstrap.min.css">
<script src="https://cdn.staticfile.org/jquery/3.5.1/jquery.min.js"></script>
<script src="https://cdn.staticfile.org/popper.js/1.16.0/umd/popper.min.js"></script>
<script src="https://cdn.staticfile.org/twitter-bootstrap/4.5.3/js/bootstrap.min.js"></script>
<style>
.trapezoid {
width: 200px;
height: 100px;
border-top: 50px solid #ccc;
border-left: 25px solid transparent;
border-right: 25px solid transparent;
position: fixed;
bottom: 0;
left: 50%;
transform: translateX(-50%) skewX(-15deg);
}
.rounded-bottom-left {
border-bottom-left-radius: 0 !important;
}
.rounded-bottom-right {
border-bottom-right-radius: 0 !important;
}
.rounded-bottom {
border-radius: 0 0 10px 10px !important;
}
</style>
</head>
<body>
<div class="trapezoid"></div>
<script>
$(document).ready(function() {
$('.trapezoid').click(function() {
$(this).toggleClass('rounded-bottom');
$('.trapezoid').toggleClass('rounded-bottom-left rounded-bottom-right');
});
});
</script>
</body>
</html>
```
这样就实现了一个底部为反圆角的梯形盒子,并且可以通过点击容器来切换底部两个角的样式。
阅读全文
相关推荐
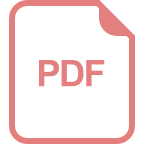
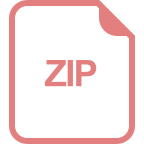

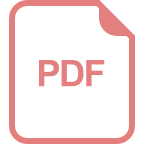
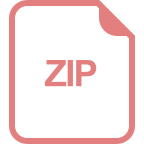
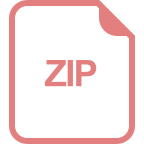
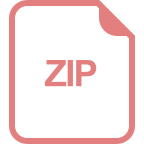
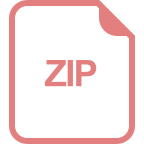
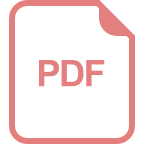
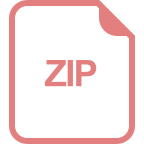
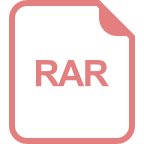
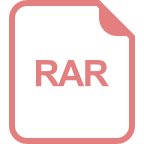
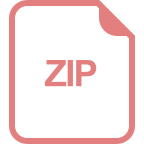
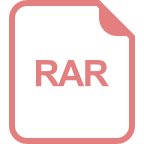
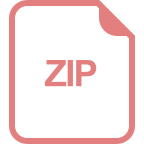
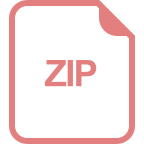