# Load model model = attempt_load(weights, map_location=device) # load FP32 model imgsz = check_img_size(imgsz, s=model.stride.max()) # check img_size if half: model.half() # to FP16 # Second-stage classifier classify = True if classify: # modelc = torch_utils.load_classifier(name='resnet101', n=2) # initialize # modelc.load_state_dict(torch.load('weights/resnet101.pt', map_location=device)['model']) # load weights modelc = LPRNet(lpr_max_len=8, phase=False, class_num=len(CHARS), dropout_rate=0).to(device) modelc.load_state_dict(torch.load('./weights/Final_LPRNet_model.pth', map_location=torch.device('cpu'))) print("load pretrained model successful!") modelc.to(device).eval()
时间: 2023-06-24 14:09:23 浏览: 187
这段代码的作用是加载模型并进行推理。首先使用`attempt_load()`函数加载FP32模型,并检查输入图片的大小。如果`half`参数为True,则将模型转换为FP16精度。接下来,判断是否需要进行第二阶段的分类操作。如果需要,就加载一个现有的分类器或者创建一个新的分类器。在这个例子中,创建了一个名为`modelc`的LPRNet模型,并加载了预训练的权重。最后,将`modelc`模型转移到设备上,并设置为评估模式。
相关问题
代码解释 # Load model model = attempt_load(weights, map_location=device) # load FP32 model stride = int(model.stride.max()) # model stride imgsz = check_img_size(imgsz, s=stride) # check img_size
这段代码的作用是加载模型(通过指定的权重文件路径),并检查模型的输入图像尺寸是否符合要求。具体解释如下:
- `attempt_load(weights, map_location=device)` 会返回一个加载了指定权重文件的模型。其中,`weights` 是指定的权重文件路径,`map_location` 是指定模型在哪个设备上运行。
- `stride = int(model.stride.max())` 用于获取模型的最大步幅(stride),并将其转换为整数类型。步幅是指模型在处理图像时,每次移动的像素数目。
- `check_img_size(imgsz, s=stride)` 用于检查输入图像的尺寸是否符合要求。其中,`imgsz` 是指定的输入图像尺寸,`s` 是模型的步幅。如果输入图像的尺寸不符合要求,则会将其调整为最接近的符合要求的尺寸。
cudnn.benchmark = True weights = 'weights/yolov5s.pt' # 模型加载路径 imgsz = 640 # 预测图尺寸大小 self.conf_thres = 0.25 # NMS置信度 self.iou_thres = 0.45 # IOU阈值 # 载入模型 self.model = attempt_load(weights, map_location=self.device) stride = int(self.model.stride.max()) self.imgsz = check_img_size(imgsz, s=stride) if self.half: self.model.half() # to FP16 # 从模型中获取各类别名称 self.names = self.model.module.names if hasattr(self.model, 'module') else self.model.names # 给每一个类别初始化颜色 self.colors = [[random.randint(0, 255) for _ in range(3)] for _ in self.names]
这部分代码用于初始化目标检测模型。首先,设置了`cudnn.benchmark = True`以提高模型的性能。然后,定义了一些变量,包括模型的权重文件路径`weights`、预测图像的尺寸`imgsz`、NMS(非极大值抑制)的置信度阈值`conf_thres`和IOU(交并比)的阈值`iou_thres`。
接下来,调用`attempt_load()`函数加载模型权重文件,并根据设备类型选择计算设备。然后,根据模型的stride值和预测图像尺寸,设置了`stride`和`imgsz`变量的值。
如果使用半精度运算(half precision),则将模型转换为FP16格式。然后,从模型中获取类别名称,并为每个类别随机初始化一个颜色。
这段代码表明了初始化目标检测模型和相关参数的过程。
阅读全文
相关推荐
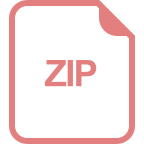
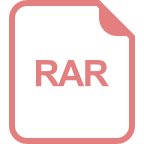
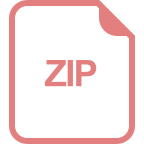

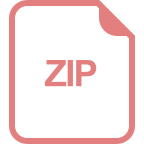
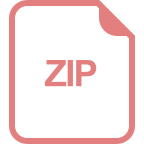
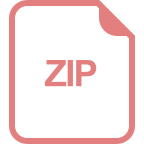