python筛选掉excel中基金经理comment为空的行
时间: 2024-04-30 20:24:48 浏览: 45
你可以使用 Pandas 库来读取 Excel 文件并进行筛选。具体步骤如下:
1. 首先,安装 Pandas 库:
```
pip install pandas
```
2. 导入 Pandas 库:
```python
import pandas as pd
```
3. 使用 Pandas 的 `read_excel` 函数读取 Excel 文件:
```python
df = pd.read_excel('filename.xlsx')
```
4. 使用 Pandas 的 `dropna` 函数删除 `comment` 列为空的行:
```python
df = df.dropna(subset=['comment'])
```
完整代码如下:
```python
import pandas as pd
# 读取 Excel 文件
df = pd.read_excel('filename.xlsx')
# 删除 comment 列为空的行
df = df.dropna(subset=['comment'])
# 输出筛选后的结果
print(df)
```
其中,`filename.xlsx` 是你要读取的 Excel 文件的文件名。
相关问题
python筛选excel中‘基金经理comment’为空的行
可以使用 `pandas` 库来读取 Excel 文件,并筛选出 `‘基金经理comment’` 列为空的行。以下是示例代码:
```python
import pandas as pd
# 读取 Excel 文件
df = pd.read_excel('your_file.xlsx')
# 筛选出 '基金经理comment' 列为空的行
df = df[df['基金经理comment'].isnull()]
# 输出结果
print(df)
```
其中,`isnull()` 方法用于判断某个元素是否为空值,返回一个布尔类型的 Series。筛选时,需要将该 Series 传递给 DataFrame 的索引,即 `df[df['基金经理comment'].isnull()]`。
python筛选掉dataframe中’基金经理comment’和‘股票名称’为空的行
可以使用 Pandas 库来实现筛选,具体代码如下:
```python
import pandas as pd
# 读取数据
df = pd.read_csv('data.csv')
# 筛选出基金经理comment和股票名称不为空的行
df = df.dropna(subset=['基金经理comment', '股票名称'])
# 输出结果
print(df)
```
其中 `dropna()` 函数可以删除含有空值的行,`subset` 参数可以指定需要筛选的列。以上代码将会输出删除空值后的数据框。
阅读全文
相关推荐
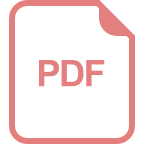
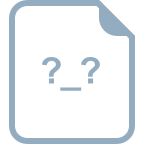














