PCL对二值化描述子进行ICP匹配
时间: 2023-08-19 20:04:57 浏览: 39
下面是一个示例代码,展示了如何使用PCL对二值化描述子进行ICP匹配:
```cpp
// 加载点云
pcl::PointCloud<pcl::PointXYZ>::Ptr cloud_src(new pcl::PointCloud<pcl::PointXYZ>);
pcl::PointCloud<pcl::PointXYZ>::Ptr cloud_tgt(new pcl::PointCloud<pcl::PointXYZ>);
pcl::io::loadPCDFile("cloud_src.pcd", *cloud_src);
pcl::io::loadPCDFile("cloud_tgt.pcd", *cloud_tgt);
// 计算二值化描述子
pcl::SHOTColorEstimation<pcl::PointXYZ, pcl::Normal, pcl::SHOT1344> shot;
shot.setInputCloud(cloud_src);
shot.setInputNormals(normals_src);
pcl::PointCloud<pcl::SHOT1344>::Ptr descriptors_src(new pcl::PointCloud<pcl::SHOT1344>);
shot.compute(*descriptors_src);
shot.setInputCloud(cloud_tgt);
shot.setInputNormals(normals_tgt);
pcl::PointCloud<pcl::SHOT1344>::Ptr descriptors_tgt(new pcl::PointCloud<pcl::SHOT1344>);
shot.compute(*descriptors_tgt);
// 使用ICP算法进行匹配
pcl::IterativeClosestPoint<pcl::PointXYZ, pcl::PointXYZ> icp;
icp.setInputSource(cloud_src);
icp.setInputTarget(cloud_tgt);
// 将二值化描述子作为ICP算法的特征输入
pcl::PointCloud<pcl::PointXYZ>::Ptr cloud_src_keypoints(new pcl::PointCloud<pcl::PointXYZ>);
pcl::PointCloud<pcl::PointXYZ>::Ptr cloud_tgt_keypoints(new pcl::PointCloud<pcl::PointXYZ>);
pcl::PointCloud<pcl::SHOT1344>::Ptr descriptors_src_keypoints(new pcl::PointCloud<pcl::SHOT1344>);
pcl::PointCloud<pcl::SHOT1344>::Ptr descriptors_tgt_keypoints(new pcl::PointCloud<pcl::SHOT1344>);
// 选取一些关键点
pcl::UniformSampling<pcl::PointXYZ> uniform_sampling;
uniform_sampling.setInputCloud(cloud_src);
uniform_sampling.setRadiusSearch(0.01f);
pcl::PointCloud<int> keypoint_indices_src;
uniform_sampling.compute(keypoint_indices_src);
pcl::copyPointCloud(*cloud_src, keypoint_indices_src.points, *cloud_src_keypoints);
pcl::copyPointCloud(*descriptors_src, keypoint_indices_src.points, *descriptors_src_keypoints);
uniform_sampling.setInputCloud(cloud_tgt);
pcl::PointCloud<int> keypoint_indices_tgt;
uniform_sampling.compute(keypoint_indices_tgt);
pcl::copyPointCloud(*cloud_tgt, keypoint_indices_tgt.points, *cloud_tgt_keypoints);
pcl::copyPointCloud(*descriptors_tgt, keypoint_indices_tgt.points, *descriptors_tgt_keypoints);
// 设置ICP算法的特征点和描述子
icp.setSourceFeatures(descriptors_src_keypoints);
icp.setTargetFeatures(descriptors_tgt_keypoints);
icp.setSourceKeypoints(cloud_src_keypoints);
icp.setTargetKeypoints(cloud_tgt_keypoints);
// 运行ICP算法
pcl::PointCloud<pcl::PointXYZ>::Ptr aligned(new pcl::PointCloud<pcl::PointXYZ>);
icp.align(*aligned);
// 输出匹配结果
if (icp.hasConverged()) {
std::cout << "ICP converged. Score: " << icp.getFitnessScore() << std::endl;
std::cout << icp.getFinalTransformation() << std::endl;
} else {
std::cout << "ICP did not converge." << std::endl;
}
```
上述代码中,首先使用SHOT描述子计算了两个点云的二值化描述子。然后,使用UniformSampling算法选取了一些关键点,并将这些关键点和对应的描述子作为ICP算法的特征点和描述子输入。最后,运行ICP算法,并输出匹配结果。
相关推荐
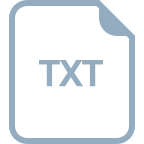
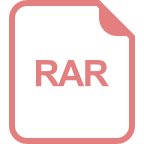














