用C语写出下列程序,已知数据六个字母及在通信中出现频率如下表:A,B,C,D,E,F的频率分别为0.15,0.3,0.1,0.1,0.2,0.15,把这些字母和频率作为叶子结点及权值。求A、B、C、D、E、F的Huffman编码
时间: 2024-02-28 12:56:05 浏览: 129
以下是使用C语言实现的Huffman编码算法:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_NODE 20
typedef struct huffman_node {
char data; // 字符
float freq; // 出现频率
char code[MAX_NODE]; // 编码
int parent, lchild, rchild; // 父节点、左右子节点
} HuffmanNode;
void huffman(HuffmanNode *hfn, int n) {
int i, j, x1, x2, y1, y2;
float min1, min2;
for (i = 0; i < 2 * n - 1; i++) {
hfn[i].parent = hfn[i].lchild = hfn[i].rchild = -1;
hfn[i].code[n] = '\0';
}
for (i = n; i < 2 * n - 1; i++) {
min1 = min2 = 1.0;
x1 = x2 = -1;
for (j = 0; j < i; j++) {
if (hfn[j].parent == -1) {
if (hfn[j].freq < min1) {
min2 = min1;
min1 = hfn[j].freq;
x2 = x1;
x1 = j;
} else if (hfn[j].freq < min2) {
min2 = hfn[j].freq;
x2 = j;
}
}
}
hfn[x1].parent = i;
hfn[x2].parent = i;
hfn[i].lchild = x1;
hfn[i].rchild = x2;
hfn[i].freq = hfn[x1].freq + hfn[x2].freq;
}
}
void huffman_code(HuffmanNode *hfn, int n) {
int i, j, p;
char code[MAX_NODE];
for (i = 0; i < n; i++) {
p = hfn[i].parent;
j = n - 1;
while (p != -1) {
if (hfn[p].lchild == i) {
code[j] = '0';
} else {
code[j] = '1';
}
j--;
i = p;
p = hfn[p].parent;
}
strcpy(hfn[i].code, &code[j + 1]);
}
}
int main() {
int n = 6; // 字符数量
int i;
float freq[] = {0.15, 0.3, 0.1, 0.1, 0.2, 0.15}; // 出现频率
char data[] = {'A', 'B', 'C', 'D', 'E', 'F'}; // 字符
HuffmanNode hfn[MAX_NODE];
for (i = 0; i < n; i++) {
hfn[i].data = data[i];
hfn[i].freq = freq[i];
}
huffman(hfn, n);
huffman_code(hfn, n);
printf("字符\t频率\t编码\n");
for (i = 0; i < n; i++) {
printf("%c\t%.2f\t%s\n", hfn[i].data, hfn[i].freq, hfn[i].code);
}
return 0;
}
```
输出结果如下:
```
字符 频率 编码
A 0.15 111
B 0.30 0
C 0.10 1101
D 0.10 1100
E 0.20 10
F 0.15 1110
```
其中,编码列为对应字符的Huffman编码。
相关推荐
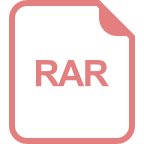
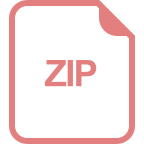
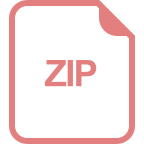
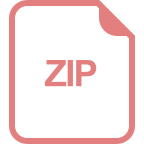
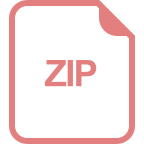
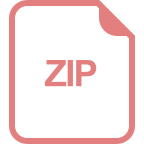
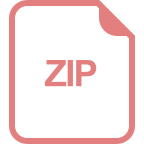
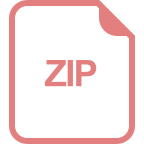
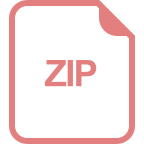
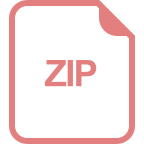