Android studio 使用Service生成随机数
时间: 2023-07-15 21:11:18 浏览: 175
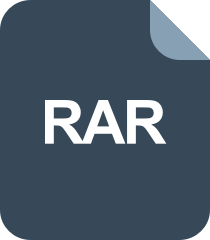
随机数生成工具
可以使用 Service 在后台生成随机数。以下是一份示例代码:
```java
public class RandomNumberService extends Service {
private static final String TAG = "RandomNumberService";
private final IBinder mBinder = new LocalBinder();
private final Random mGenerator = new Random();
@Override
public void onCreate() {
Log.d(TAG, "onCreate");
}
@Override
public int onStartCommand(Intent intent, int flags, int startId) {
Log.d(TAG, "onStartCommand");
return START_NOT_STICKY;
}
@Override
public IBinder onBind(Intent intent) {
Log.d(TAG, "onBind");
return mBinder;
}
public class LocalBinder extends Binder {
RandomNumberService getService() {
return RandomNumberService.this;
}
}
public int getRandomNumber() {
return mGenerator.nextInt();
}
}
```
然后在你的 Activity 中,通过启动 Service 和绑定 Service 的方式获取随机数:
```java
public class MainActivity extends AppCompatActivity {
private boolean mBound = false;
private RandomNumberService mService;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
Intent intent = new Intent(this, RandomNumberService.class);
startService(intent);
bindService(intent, mConnection, Context.BIND_AUTO_CREATE);
}
@Override
protected void onDestroy() {
super.onDestroy();
if (mBound) {
unbindService(mConnection);
mBound = false;
}
}
private ServiceConnection mConnection = new ServiceConnection() {
@Override
public void onServiceConnected(ComponentName componentName, IBinder iBinder) {
RandomNumberService.LocalBinder binder = (RandomNumberService.LocalBinder) iBinder;
mService = binder.getService();
mBound = true;
}
@Override
public void onServiceDisconnected(ComponentName componentName) {
mBound = false;
}
};
public void onGetRandomNumber(View view) {
if (mBound) {
int randomNumber = mService.getRandomNumber();
Toast.makeText(this, "Random number: " + randomNumber, Toast.LENGTH_SHORT).show();
}
}
}
```
其中 `onGetRandomNumber` 是一个按钮的点击事件,当用户点击按钮时,会调用 `getRandomNumber` 方法获取随机数,并通过 Toast 显示出来。
阅读全文
相关推荐
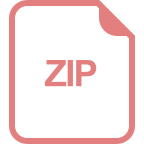
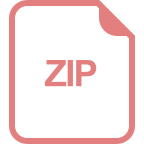
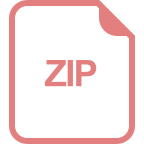
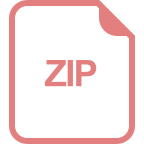
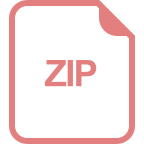
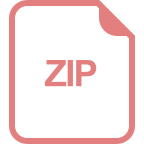
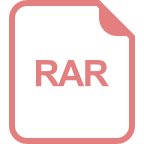
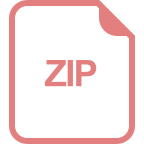
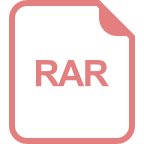
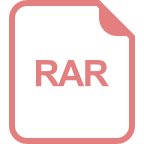
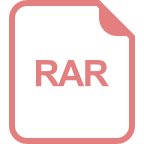
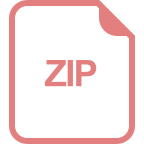
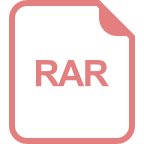
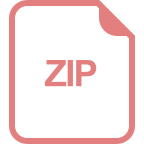
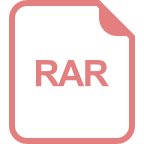
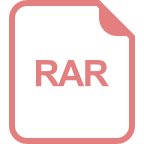