输入一串电文统计出现频次并输出哈夫曼编码
时间: 2023-04-27 15:01:04 浏览: 106
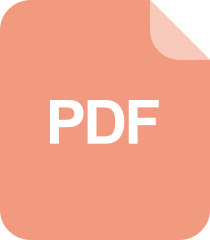
【C++】根据输入的字符串生成哈夫曼树, 并进行哈夫曼编码和解码

好的,下面是中文回复:
要统计一串电文中每个字符出现的频次,可以先遍历一遍这个字符串,用一个字典记录每个字符出现的次数。然后,可以根据这个字典构建一个哈夫曼树,生成每个字符的哈夫曼编码。
哈夫曼编码是一种前缀编码,即每个字符的编码都不是其他字符编码的前缀。这样可以保证在解码时不会出现歧义。构建哈夫曼树的过程可以使用贪心算法,每次选择频次最小的两个节点合并,直到只剩下一个节点为止。
最后,可以根据哈夫曼树生成每个字符的编码。从根节点开始,遍历到每个叶子节点,记录下路径上的编码即可。最终输出每个字符的频次和哈夫曼编码。
阅读全文
相关推荐
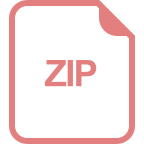
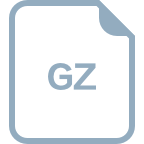















