using JetBrains.Annotations; using System.Collections; using System.Collections.Generic; using UnityEngine; public class NewBehaviourScript : MonoBehaviour { private Transform firePoint; private GameObject bulletPrefab; private Rigidbody2D rb; float speed = 5.0f; float jump = 300.0f; void Start() { firePoint = transform.Find("Player/Firepoint"); bulletPrefab = (GameObject) Resources.Load("Prefabs/Bullet"); rb = GetComponent<Rigidbody2D>(); Debug.Log(bulletPrefab); } void Update() { //左右移动 float hor = Input.GetAxis("Horizontal"); move(hor); //跳跃 if (Input.GetKeyDown(KeyCode.Space)) { rb.AddForce(Vector2.up * jump); } //射击? if (Input.GetMouseButtonDown(0)) { GameObject bullet = Instantiate(bulletPrefab, firePoint.position, Quaternion.identity); bullet.GetComponent<Bullet>().setMoveDir(transform.forward); } } private void move(float hor) { transform.Translate(hor * Time.deltaTime * speed, 0, 0); } }
时间: 2024-02-14 10:26:12 浏览: 24
你的代码看起来基本上是正确的,但有一个小问题在于你调用了`bullet.GetComponent<Bullet>().setMoveDir(transform.forward);`,但是在Bullet脚本中并没有一个名为setMoveDir的方法。你需要根据之前的建议,在Bullet脚本中添加一个公共方法来设置移动方向。
以下是修改后的代码示例:
```csharp
using UnityEngine;
public class Bullet : MonoBehaviour
{
private Vector3 moveDirection;
public void SetMoveDir(Vector3 direction)
{
moveDirection = direction.normalized;
}
void Update()
{
transform.position += moveDirection * Time.deltaTime;
}
}
```
在Bullet脚本中,我们添加了SetMoveDir方法来设置子弹的移动方向。
接下来,在PlayerController脚本中,你可以使用`bullet.GetComponent<Bullet>().SetMoveDir(transform.forward);`来设置子弹的移动方向。
希望这次能帮到你!如果还有其他问题,请随时提问。
相关问题
java: 程序包org.jetbrains.annotations不存在的解决方法
这个问题一般是由于缺少 JetBrains 的注释库导致的。您可以通过以下几种方法来解决这个问题:
1. 在您的项目中添加 JetBrains 的注释库依赖。您可以在 Maven 中添加以下依赖项:
```xml
<dependency>
<groupId>org.jetbrains</groupId>
<artifactId>annotations</artifactId>
<version>17.0.0</version>
</dependency>
```
2. 如果您正在使用 IntelliJ IDEA 进行开发,您可以尝试更新您的 IDE 版本。有时,较旧的版本可能会遇到这种问题。
3. 您还可以尝试在您的代码中忽略这些注释。例如,如果您在使用 @NotNull 注释时遇到了问题,您可以尝试使用以下注释代替:
```java
@SuppressWarnings("NullableProblems")
```
希望这些方法能够帮助您解决问题。如果您还有其他问题,请随时问我。
Cannot resolve org.jetbrains:annotations:0.0.1-SNAPSHOT
这个错误通常表示你的项目中缺少 JetBrains 注解库的依赖。你可以通过在项目的 build.gradle 文件中添加以下代码来解决这个问题:
```
dependencies {
compile 'org.jetbrains:annotations:15.0'
}
```
如果你使用的是 Kotlin,可以使用以下代码:
```
dependencies {
compile "org.jetbrains.kotlin:kotlin-stdlib-jdk8"
compile "org.jetbrains:annotations:15.0"
}
```
这样就可以解决这个错误了。
相关推荐
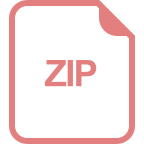
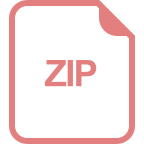
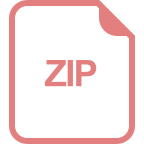












