使用socke技术编写一段代码,使用c++代码,使用socket技术完成获取本地主机的IP地址、子网掩码、网络关地址、域名服务器地址等信息的功能
时间: 2024-02-07 21:01:54 浏览: 112
下面是一个使用C++编写的获取本地主机信息的示例代码:
```c++
#include <iostream>
#include <string.h>
#include <sys/socket.h>
#include <arpa/inet.h>
#include <ifaddrs.h>
#include <netdb.h>
#include <unistd.h>
using namespace std;
// 获取本地主机名和IP地址
void get_host_ip(char* ip) {
int fd;
struct ifaddrs *ifa, *ifa_tmp;
struct sockaddr_in *sin;
char buf[INET_ADDRSTRLEN];
if (getifaddrs(&ifa) == -1) {
perror("getifaddrs");
return;
}
for (ifa_tmp = ifa; ifa_tmp != NULL; ifa_tmp = ifa_tmp->ifa_next) {
if (ifa_tmp->ifa_addr == NULL) {
continue;
}
if ((ifa_tmp->ifa_flags & IFF_UP) == 0) {
continue;
}
if (ifa_tmp->ifa_addr->sa_family != AF_INET) {
continue;
}
sin = (struct sockaddr_in *)ifa_tmp->ifa_addr;
inet_ntop(AF_INET, &(sin->sin_addr), buf, INET_ADDRSTRLEN);
if (strcmp(ifa_tmp->ifa_name, "lo") != 0) {
strcpy(ip, buf);
break;
}
}
freeifaddrs(ifa);
}
// 获取本地主机的子网掩码
void get_netmask(char* netmask) {
int fd;
struct ifreq ifr;
fd = socket(AF_INET, SOCK_DGRAM, 0);
if (fd < 0) {
perror("socket");
return;
}
strcpy(ifr.ifr_name, "eth0");
if (ioctl(fd, SIOCGIFNETMASK, &ifr) == -1) {
perror("ioctl");
close(fd);
return;
}
inet_ntop(AF_INET, &(reinterpret_cast<struct sockaddr_in*>(&ifr.ifr_addr)->sin_addr), netmask, INET_ADDRSTRLEN);
close(fd);
}
// 获取本地主机的网关地址
void get_gateway(char* gateway) {
FILE *fp;
char buf[256];
char *iface, *dest, *gw;
fp = fopen("/proc/net/route", "r");
if (fp == NULL) {
perror("fopen");
return;
}
while (fgets(buf, sizeof(buf), fp)) {
iface = strtok(buf, " \t");
dest = strtok(NULL, " \t");
gw = strtok(NULL, " \t");
if (iface != NULL && dest != NULL && strcmp(dest, "00000000") == 0 && gw != NULL) {
unsigned long addr = strtoul(gw, NULL, 16);
struct in_addr inaddr;
inaddr.s_addr = htonl(addr);
strcpy(gateway, inet_ntoa(inaddr));
break;
}
}
fclose(fp);
}
// 获取本地主机的DNS服务器地址
void get_nameservers(char* nameservers) {
FILE *fp;
char buf[256];
char *p;
fp = fopen("/etc/resolv.conf", "r");
if (fp == NULL) {
perror("fopen");
return;
}
while (fgets(buf, sizeof(buf), fp)) {
if (strncmp(buf, "nameserver", strlen("nameserver")) == 0) {
p = strtok(buf, " \t\n");
if (p != NULL) {
p = strtok(NULL, " \t\n");
if (p != NULL) {
strcat(nameservers, p);
strcat(nameservers, " ");
}
}
}
}
fclose(fp);
}
int main() {
char hostname[256];
char ip_address[INET_ADDRSTRLEN];
char netmask[INET_ADDRSTRLEN];
char gateway[INET_ADDRSTRLEN];
char nameservers[256] = "";
gethostname(hostname, sizeof(hostname));
get_host_ip(ip_address);
get_netmask(netmask);
get_gateway(gateway);
get_nameservers(nameservers);
cout << "Hostname: " << hostname << endl;
cout << "IP Address: " << ip_address << endl;
cout << "Netmask: " << netmask << endl;
cout << "Gateway: " << gateway << endl;
cout << "Nameservers: " << nameservers << endl;
return 0;
}
```
需要注意的是,本代码中获取子网掩码和网关地址的方式是基于Linux系统的,如果您是在其他操作系统上运行此代码,可能需要根据不同的系统做出相应的修改。
阅读全文
相关推荐








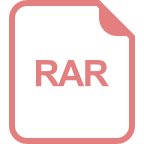

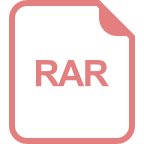







