java中编写一个程序,保存一个包含整数、日期和地址的对象到文件sample.dat中,并从该文件中读取对象显示出来。
时间: 2023-06-10 19:04:08 浏览: 135
好的,我可以为您解决这个问题。您可以按照以下步骤来实现:
1. 创建一个包含整数、日期和地址的类,例如:
```
public class ObjectToFile {
private int number;
private Date date;
private String address;
public ObjectToFile(int number, Date date, String address) {
this.number = number;
this.date = date;
this.address = address;
}
// 省略 getter 和 setter 方法
}
```
2. 在该类中实现 Serializable 接口,以便对象可以被序列化和反序列化:
```
public class ObjectToFile implements Serializable {
// 省略类定义和构造方法
// 序列化实现
private void writeObject(ObjectOutputStream out) throws IOException {
out.writeInt(number);
out.writeObject(date);
out.writeUTF(address);
}
// 反序列化实现
private void readObject(ObjectInputStream in) throws IOException, ClassNotFoundException {
number = in.readInt();
date = (Date)in.readObject();
address = in.readUTF();
}
}
```
3. 在主程序中,创建一个 ObjectToFile 对象并将其保存到文件中:
```
public static void main(String[] args) throws Exception {
ObjectToFile obj = new ObjectToFile(123, new Date(), "Sample Address");
FileOutputStream fileOut = new FileOutputStream("sample.dat");
ObjectOutputStream objOut = new ObjectOutputStream(fileOut);
objOut.writeObject(obj);
objOut.close();
}
```
4. 从文件中读取对象并显示它:
```
public static void main(String[] args) throws Exception {
FileInputStream fileIn = new FileInputStream("sample.dat");
ObjectInputStream objIn = new ObjectInputStream(fileIn);
ObjectToFile obj = (ObjectToFile)objIn.readObject();
System.out.println("Number: " + obj.getNumber());
System.out.println("Date: " + obj.getDate());
System.out.println("Address: " + obj.getAddress());
objIn.close();
}
```
这些步骤可以帮助您将包含整数、日期和地址的对象保存到文件中,并从文件中读取对象并显示它。希望可以帮到您。
相关推荐
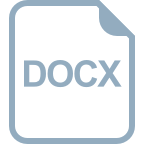
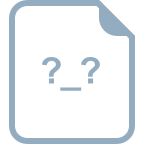














