java消息通知实现代码
时间: 2023-11-27 07:02:34 浏览: 52
以下是基于Java实现消息通知的示例代码:
1. 消息类定义
```java
public class Message {
private String title;
private String content;
private Date time;
// 省略getter和setter方法
}
```
2. 消息通知接口定义
```java
public interface Notification {
void send(Message message);
}
```
3. 邮件通知实现类
```java
public class EmailNotification implements Notification {
private String smtpHost;
private String username;
private String password;
public EmailNotification(String smtpHost, String username, String password) {
this.smtpHost = smtpHost;
this.username = username;
this.password = password;
}
public void send(Message message) {
// 使用JavaMail发送邮件
Properties props = new Properties();
props.put("mail.smtp.host", smtpHost);
props.put("mail.smtp.auth", "true");
Authenticator authenticator = new Authenticator() {
protected PasswordAuthentication getPasswordAuthentication() {
return new PasswordAuthentication(username, password);
}
};
Session session = Session.getInstance(props, authenticator);
try {
MimeMessage mimeMessage = new MimeMessage(session);
mimeMessage.setFrom(new InternetAddress(username));
mimeMessage.setRecipient(Message.RecipientType.TO,
new InternetAddress("recipient@example.com"));
mimeMessage.setSubject(message.getTitle());
mimeMessage.setText(message.getContent());
Transport.send(mimeMessage);
} catch (MessagingException e) {
e.printStackTrace();
}
}
}
```
4. 短信通知实现类
```java
public class SmsNotification implements Notification {
private String apiKey;
private String apiSecret;
public SmsNotification(String apiKey, String apiSecret) {
this.apiKey = apiKey;
this.apiSecret = apiSecret;
}
public void send(Message message) {
// 使用第三方短信服务发送短信
MessageClient client = new MessageClient(apiKey, apiSecret);
MessageResponse response = client.send("recipient_phone_number", message.getContent());
if (response.getStatusCode() == 200) {
System.out.println("短信发送成功");
} else {
System.out.println("短信发送失败");
}
}
}
```
以上是邮件通知和短信通知的实现示例,可以根据自己的需求和场景进行适当修改。在实际应用中,可以根据需要使用不同的通知方式,例如系统通知、推送通知等。
相关推荐
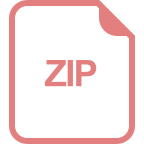
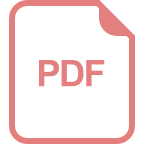
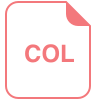
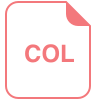
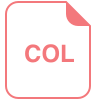
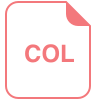
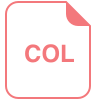









