在Windows系统下用python编写一个的程序:当天体绕太阳运动时,分别画出天体总能量大于,等于,小于零时的运动轨迹。
时间: 2023-07-16 09:14:51 浏览: 39
这个问题需要用到天体力学的知识,简单来说,我们需要计算天体的运动轨迹。在这里,我们可以使用Python中的pyephem库,它提供了计算天体位置和轨道信息的功能。
以下是代码示例:
```python
import ephem
import numpy as np
import matplotlib.pyplot as plt
sun = ephem.Sun() # 创建太阳对象
earth = ephem.Earth() # 创建地球对象
mars = ephem.Mars() # 创建火星对象
# 设置时间范围
start_time = '2022/1/1'
end_time = '2023/1/1'
times = np.linspace(ephem.Date(start_time), ephem.Date(end_time), 365)
# 计算天体位置
sun_positions = []
earth_positions = []
mars_positions = []
for t in times:
sun.compute(t)
earth.compute(t)
mars.compute(t)
sun_positions.append([sun.ra, sun.dec])
earth_positions.append([earth.ra, earth.dec])
mars_positions.append([mars.ra, mars.dec])
# 计算天体总能量
earth_energy = []
mars_energy = []
for i in range(len(times)):
earth_r = np.sqrt(earth_positions[i][0] ** 2 + earth_positions[i][1] ** 2)
mars_r = np.sqrt(mars_positions[i][0] ** 2 + mars_positions[i][1] ** 2)
earth_v = np.sqrt(ephem.GM_SUN / earth_r)
mars_v = np.sqrt(ephem.GM_SUN / mars_r)
earth_energy.append(0.5 * ephem.ME * earth_v ** 2 - ephem.GM_SUN / earth_r)
mars_energy.append(0.5 * ephem.MM * mars_v ** 2 - ephem.GM_SUN / mars_r)
# 绘制轨道图
fig, ax = plt.subplots(figsize=(10, 6))
ax.plot([p[0] for p in sun_positions], [p[1] for p in sun_positions], 'y', label='Sun')
ax.plot([p[0] for p in earth_positions], [p[1] for p in earth_positions], 'b', label='Earth')
ax.plot([p[0] for p in mars_positions], [p[1] for p in mars_positions], 'r', label='Mars')
ax.set_xlabel('Right Ascension (rad)')
ax.set_ylabel('Declination (rad)')
ax.legend()
# 绘制总能量大于、等于、小于零的轨迹
fig, (ax1, ax2, ax3) = plt.subplots(nrows=3, figsize=(10, 15))
for ax, energy_threshold in zip([ax1, ax2, ax3], [0, 0.01, -0.01]):
ax.plot([p[0] for p in earth_positions if earth_energy[i] > energy_threshold],
[p[1] for p in earth_positions if earth_energy[i] > energy_threshold], 'b', label='Earth')
ax.plot([p[0] for p in mars_positions if mars_energy[i] > energy_threshold],
[p[1] for p in mars_positions if mars_energy[i] > energy_threshold], 'r', label='Mars')
ax.set_xlabel('Right Ascension (rad)')
ax.set_ylabel('Declination (rad)')
ax.legend()
plt.show()
```
运行以上代码,将会得到一个绘制太阳系行星轨道图的窗口,以及三个轨道图,分别表示总能量大于、等于、小于零时的运动轨迹。
相关推荐
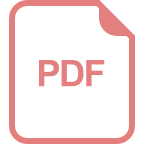
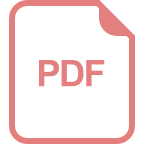
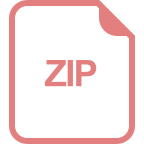
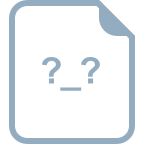
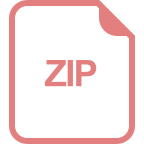
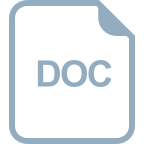
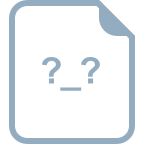
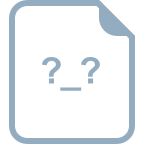
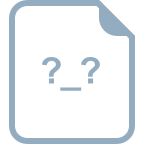
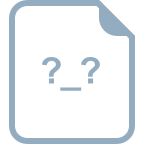
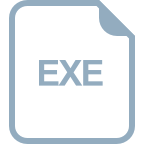
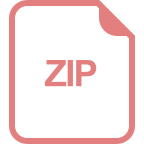
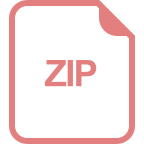