怎么在mainwindow.cpp中使用其他界面上的控件
时间: 2024-04-10 07:28:18 浏览: 40
要在MainWindow.cpp中使用其他界面上的控件,你需要先获取对这些控件的指针,然后就可以通过指针来访问和操作这些控件。
一种常见的方法是在MainWindow类的构造函数中传递指向其他界面的指针。假设你的其他界面是AnotherWindow,你可以在MainWindow.cpp中这样做:
```cpp
#include "mainwindow.h"
#include "anotherwindow.h"
MainWindow::MainWindow(QWidget *parent)
: QMainWindow(parent)
{
// 创建 AnotherWindow 对象
AnotherWindow *anotherWindow = new AnotherWindow(this);
// 获取指向控件的指针
QPushButton *button = anotherWindow->getButton();
// 对控件进行操作
button->setText("Hello World");
// ...
}
```
在上面的代码中,我们假设AnotherWindow类有一个公共的getButton()函数,返回指向按钮控件的指针。然后我们在MainWindow构造函数中获取这个指针,并对按钮进行操作。
请注意,这只是一种常见的方法,具体实现可能会因你的代码结构而有所不同。你需要根据你的实际情况来确定如何传递指针和访问控件。
相关问题
在QT界面设计中,在mainwindow环境下,使用一个label控件名叫22的进行实时时间显示,帮我写出mainwindow.cpp和头文件的代码,使这个控件能够显示实时时间,控件22已经在界面文件里面拖入了,帮我写出代码
要在Qt的`mainwindow.cpp`和相应的头文件中实现实时时间显示,你需要做以下几个步骤:
**mainwindow.h(头文件)**:
```cpp
#ifndef MAINWINDOW_H
#define MAINWINDOW_H
#include <QObject>
#include <QLabel>
class QLabel* QLabel22; // 声明全局指针,用于后续引用
class MainWindow : public QMainWindow
{
Q_OBJECT
public:
explicit MainWindow(QWidget *parent = nullptr);
~MainWindow();
private slots:
void updateTime(); // 定义更新时间的槽函数
signals:
void timeChanged(const QString& currentTime); // 发送时间变化信号
private:
QTimer *timeTimer; // 定时器实例
};
#endif // MAINWINDOW_H
```
**mainwindow.cpp (主体文件)**:
```cpp
#include "mainwindow.h"
// 在mainwindow.cpp的开头包含头文件
#include "mainwindow.h"
MainWindow::MainWindow(QWidget *parent)
: QMainWindow(parent)
{
// 在构造函数中初始化label22并设置槽函数
QLabel22 = new QLabel(tr("实时时间"), this); // 获取已存在的label22实例
connect(timeTimer, &QTimer::timeout, this, &MainWindow::updateTime); // 连接定时器信号到槽函数
// 创建定时器并启动
timeTimer = new QTimer(this);
connect(timeTimer, &QTimer::timeout, this, &MainWindow::timeChanged);
timeTimer->start(1000); // 每秒更新一次时间
}
void MainWindow::updateTime()
{
QDateTime currentDateTime = QDateTime::currentDateTime();
QString currentTime = currentDateTime.toString("hh:mm:ss"); // 格式化时间为小时:分钟:秒
emit timeChanged(currentTime); // 通过信号更新label的时间
QLabel22->setText(currentTime); // 设置label的内容
}
MainWindow::~MainWindow() {
delete timeTimer;
}
```
上述代码定义了一个`MainWindow`类,其中包含一个定时器`timeTimer`,每秒钟调用`updateTime()`函数。`updateTime()`会获取当前时间并将其格式化为字符串,然后通过`emit timeChanged()`信号通知外部组件(在这里是`mainwindow`),最后更新`QLabel22`的文本。
在QT界面设计中,在mainwindow环境下,使用一个label控件名叫label_20的进行实时时间显示,帮我写出mainwindow.cpp和头文件的代码,使这个控件能够显示实时时间,控件22已经在界面文件里面拖入了,帮我写出代码
要在Qt中创建一个名为`label_20`的实时时间显示QLabel,你需要在`mainwindow.h`头文件中声明该控件,然后在`mainwindow.cpp`文件中实现更新时间的功能。下面是代码示例:
**mainwindow.h**
```cpp
#ifndef MAINWINDOW_H
#define MAINWINDOW_H
#include <QObject>
#include <QLabel>
class MainWindow : public QMainWindow
{
Q_OBJECT
public:
explicit MainWindow(QWidget *parent = nullptr);
~MainWindow();
private slots:
void updateTime();
private:
QLabel *label_20; // 声明QLabel实例
};
#endif // MAINWINDOW_H
```
**mainwindow.cpp**
```cpp
#include "mainwindow.h"
#include <QTimer> // 引入QTimer用于定时刷新时间
// 实现MainWindow构造函数
MainWindow::MainWindow(QWidget *parent)
: QMainWindow(parent)
{
label_20 = new QLabel("实时时间", this); // 创建并设置初始标签内容
label_20->setObjectName(QStringLiteral("label_20")); // 给控件命名以便后续操作
label_20->move(10, 10); // 设置位置,具体位置根据界面布局调整
// 定义定时器以每秒更新一次时间
QTimer *timer = new QTimer(this);
connect(timer, &QTimer::timeout, this, &MainWindow::updateTime);
timer->start(1000); // 每1000毫秒(即1秒)触发一次事件
}
// 更新时间的方法
void MainWindow::updateTime()
{
QDateTime currentTime = QDateTime::currentDateTime();
QString formattedTime = currentTime.toString(QStringLiteral("hh:mm:ss")); // 格式化时间
label_20->setText(formattedTime); // 更新QLabel的内容
}
// 主窗口析构函数
MainWindow::~MainWindow()
{
delete timer;
}
```
这样,`label_20`控件就会在主线程中每秒更新显示当前的时间。记得根据实际界面布局调整`move()`方法中的坐标参数。
阅读全文
相关推荐




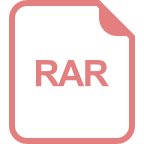











