springboot使用ip连接打印机并打印文件
时间: 2024-01-30 14:04:04 浏览: 34
要使用Spring Boot连接并打印文件,可以使用Java中的PrinterJob类。以下是一个示例代码,演示如何使用IP地址连接到打印机并打印文件:
```java
import javax.print.*;
import javax.print.attribute.HashPrintRequestAttributeSet;
import javax.print.attribute.PrintRequestAttributeSet;
import javax.print.attribute.standard.MediaSizeName;
import javax.print.attribute.standard.PrinterName;
import java.io.*;
public class PrintServiceExample {
public static void main(String[] args) throws IOException, PrintException {
// 设置打印机名称和IP地址
String printerName = "My Printer";
String ipAddress = "192.168.1.100";
String printerURI = "socket://" + ipAddress + ":9100";
// 获取打印服务
PrintService[] services = PrinterJob.lookupPrintServices();
PrintService myPrinter = null;
for (PrintService service : services) {
if (service.getName().equalsIgnoreCase(printerName)) {
myPrinter = service;
break;
}
}
// 如果找不到打印机,则创建新的打印机服务
if (myPrinter == null) {
AttributeSet attrSet = new HashAttributeSet();
attrSet.add(new PrinterName(printerName, null));
attrSet.add(new HashPrintServiceAttributeSet(new PrinterURI(printerURI)));
myPrinter = new MyPrintService(attrSet);
}
// 获取要打印的文件
String filePath = "path/to/my/file.pdf";
InputStream inputStream = new FileInputStream(new File(filePath));
// 创建打印任务
DocFlavor flavor = DocFlavor.INPUT_STREAM.AUTOSENSE;
Doc doc = new SimpleDoc(inputStream, flavor, null);
DocPrintJob job = myPrinter.createPrintJob();
// 设置打印属性
PrintRequestAttributeSet attributes = new HashPrintRequestAttributeSet();
attributes.add(MediaSizeName.ISO_A4);
// 执行打印任务
job.print(doc, attributes);
// 关闭输入流
inputStream.close();
}
}
```
在上面的示例中,我们首先设置打印机名称和IP地址。然后,我们使用PrinterJob类查找可用的打印服务。如果找不到指定名称的打印机,则创建新的打印机服务。接下来,我们获取要打印的文件,并使用DocFlavor类创建一个打印任务。最后,我们执行打印任务并关闭输入流。
请注意,上面的代码示例仅用于演示目的。实际应用中,您需要根据您的实际需求进行修改。
相关推荐
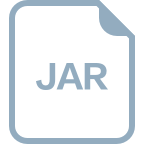
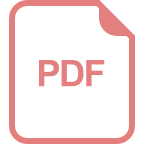














