C plus plus 开发中,临时文件名如果不唯一会造成什么后果?请举代码示例一一说明
时间: 2023-09-14 22:05:55 浏览: 60
### 回答1:
C++ 中如果使用重复的临时文件名,可能会造成文件覆盖的后果。这意味着,如果一个程序使用相同的临时文件名创建了文件,而另一个程序也使用相同的临时文件名创建了文件,那么第二个程序将会覆盖第一个程序创建的文件。
代码示例:
```
#include <fstream>
int main() {
std::ofstream file1("temp.txt");
file1 << "This is the first temporary file." << std::endl;
file1.close();
std::ofstream file2("temp.txt");
file2 << "This is the second temporary file." << std::endl;
file2.close();
return 0;
}
```
在上面的代码中,第一个程序创建了一个名为“temp.txt”的文件并写入了一些数据。第二个程序也创建了一个名为“temp.txt”的文件,但是由于文件名重复,第二个程序将覆盖第一个程序创建的文件,最终文件中只有"This is the second temporary file."这行数据.
为了避免这种情况,可以使用唯一的临时文件名或在指定文件名之前添加一些随机字符。
```
std::ofstream file1(std::tmpnam(nullptr));
std::ofstream file2(std::tmpnam(nullptr));
```
### 回答2:
在C++开发中,如果临时文件名不唯一,可能会造成以下几个后果:
1. 文件覆盖:如果多个进程或线程同时使用相同的临时文件名,并且尝试写入不同的内容,会导致文件覆盖。这将导致数据丢失或混乱。
示例代码:
```cpp
#include <fstream>
int main() {
std::ofstream tempFile("temp.txt"); // 创建一个临时文件
// 进程1
std::ofstream process1("temp.txt"); // 使用相同的临时文件名创建文件
process1 << "Process 1 data" << std::endl;
// 进程2
std::ofstream process2("temp.txt"); // 使用相同的临时文件名创建文件
process2 << "Process 2 data" << std::endl;
return 0;
}
```
上述代码中,进程1和进程2都尝试使用相同的临时文件名“temp.txt”创建文件,并向该文件写入不同的数据。由于临时文件名不唯一,第二个进程的创建会导致第一个进程创建的临时文件被覆盖,结果只会保留进程2的数据。
2. 文件读取错误:如果在处理过程中,多个线程或进程尝试同时读取相同的临时文件,可能会导致读取错误或混淆。
示例代码:
```cpp
#include <fstream>
#include <iostream>
#include <string>
#include <thread>
void readTempFile(const std::string& fileName) {
std::ifstream tempFile(fileName);
std::string line;
while (std::getline(tempFile, line)) {
// 模拟处理临时文件内容的代码
std::cout << line << std::endl;
}
}
int main() {
std::string tempFileName = "temp.txt";
std::ofstream tempFile(tempFileName); // 创建一个临时文件
tempFile << "Temporary file data" << std::endl;
// 创建两个线程尝试同时读取相同的临时文件
std::thread thread1(readTempFile, tempFileName);
std::thread thread2(readTempFile, tempFileName);
thread1.join();
thread2.join();
return 0;
}
```
上述代码中,两个线程都尝试同时读取相同的临时文件“temp.txt”。由于临时文件名不唯一,可能会导致同时打开相同文件的冲突,结果可能是文件数据读取错误或丢失。
综上所述,临时文件名不唯一可能导致文件覆盖或文件读取错误。为了避免这些问题,在开发中应该设计一套唯一的临时文件命名规则,以确保每个临时文件都有独特的名称。
### 回答3:
在C++开发中,如果临时文件名不唯一,会对文件处理和程序逻辑造成一些后果。以下是一个代码示例来说明这个问题:
```cpp
#include <iostream>
#include <fstream>
#include <cstdlib>
void writeToTempFile(std::string data) {
// 生成一个随机的临时文件名
std::string tempFileName = "tempfile.tmp"; // 临时文件名为"tempfile.tmp"
// 打开并写入数据到临时文件
std::ofstream tempFile(tempFileName);
tempFile << data;
tempFile.close();
}
int main() {
std::string inputData = "Hello, World!";
std::cout << "输入的数据:" << inputData << std::endl;
// 写入数据到临时文件
writeToTempFile(inputData);
// 尝试读取临时文件
std::ifstream tempFile("tempfile.tmp");
std::string readData;
getline(tempFile, readData);
tempFile.close();
std::cout << "读取的数据:" << readData << std::endl;
// 删除临时文件
std::remove("tempfile.tmp");
return 0;
}
```
以上代码中,我们使用了临时文件名"tempfile.tmp"来存储数据。假设我们同时运行了两个这样的程序实例,它们在同一个目录下生成临时文件。由于文件名不唯一,可能会导致以下后果:
1. 文件冲突:当两个或多个程序实例同时运行时,它们会尝试使用相同的临时文件名来创建文件。这将导致文件冲突,其中一个程序无法创建文件或覆盖了另一个程序的文件。
2. 数据丢失:如果多个程序实例都尝试读取和写入同一个临时文件,它们可能会相互干扰,导致数据丢失或损坏。
3. 文件泄漏:如果程序实例在执行过程中意外终止,例如在写入数据之后但在删除临时文件之前崩溃,则可能会导致临时文件无法删除,导致文件泄漏。
因此,为了避免这些问题,开发中临时文件名应该是唯一的,例如可以使用动态生成的随机字符串来作为临时文件名,确保每个程序实例都有自己的独立临时文件。
相关推荐
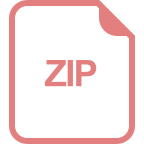
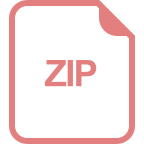














