stm32,esp8266mod发送信息在串口显示
时间: 2023-12-04 07:06:18 浏览: 131
在STM32上发送信息并在串口上显示,可以通过以下步骤实现:
1. 在STM32的代码中引入串口通信的库文件,例如"stm32f10x_usart.h"。
2. 在代码中初始化串口通信的参数,例如波特率、数据位、停止位等。
3. 在代码中配置GPIO引脚,使其与串口通信相关的引脚功能相对应。
4. 在代码中使用发送函数实现数据的发送。例如,使用USART_SendData()函数发送数据。
5. 在代码中使用串口中断函数处理接收到的数据,并在串口上显示。例如,使用USART_ITConfig()函数开启中断,并在中断服务函数中使用USART_ReceiveData()函数接收数据,然后使用printf()函数将接收到的数据输出到串口上。
以下是一个简单的例子,以USART1为例:
```
#include "stm32f10x.h"
#include <stdio.h>
void USART1_Configuration(void)
{
USART_InitTypeDef USART_InitStructure;
GPIO_InitTypeDef GPIO_InitStructure;
NVIC_InitTypeDef NVIC_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA | RCC_APB2Periph_USART1, ENABLE);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_9;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOA, &GPIO_InitStructure);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_10;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN_FLOATING;
GPIO_Init(GPIOA, &GPIO_InitStructure);
USART_InitStructure.USART_BaudRate = 115200;
USART_InitStructure.USART_WordLength = USART_WordLength_8b;
USART_InitStructure.USART_StopBits = USART_StopBits_1;
USART_InitStructure.USART_Parity = USART_Parity_No;
USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStructure.USART_Mode = USART_Mode_Rx | USART_Mode_Tx;
USART_Init(USART1, &USART_InitStructure);
NVIC_InitStructure.NVIC_IRQChannel = USART1_IRQn;
NVIC_InitStructure.NVIC_IRQChannelPreemptionPriority = 0;
NVIC_InitStructure.NVIC_IRQChannelSubPriority = 0;
NVIC_InitStructure.NVIC_IRQChannelCmd = ENABLE;
NVIC_Init(&NVIC_InitStructure);
USART_ITConfig(USART1, USART_IT_RXNE, ENABLE);
USART_Cmd(USART1, ENABLE);
}
void USART1_IRQHandler(void)
{
if (USART_GetITStatus(USART1, USART_IT_RXNE) != RESET)
{
char ch = USART_ReceiveData(USART1);
printf("%c", ch);
}
}
void USART1_SendChar(char ch)
{
while (USART_GetFlagStatus(USART1, USART_FLAG_TXE) == RESET);
USART_SendData(USART1, ch);
}
void USART1_SendString(char *str)
{
while (*str)
{
USART1_SendChar(*str++);
}
}
int main(void)
{
USART1_Configuration();
while (1)
{
USART1_SendString("Hello, world!\r\n");
delay_ms(1000);
}
}
```
在以上例子中,串口的波特率为115200,使用printf()函数将接收到的数据输出到串口上。在主函数中,使用USART1_SendString()函数发送"Hello, world!\r\n",并延时1秒。USART1_IRQHandler()为串口中断服务函数,处理接收到的数据并输出到串口上。
需要注意的是,以上代码仅为示例,实际应用中需要根据具体需求进行修改。
阅读全文
相关推荐
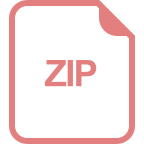
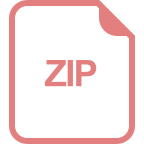
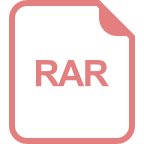
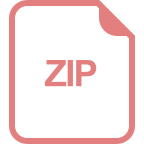
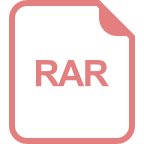
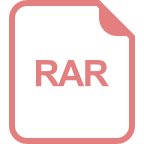
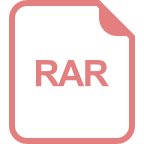
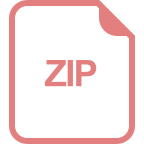
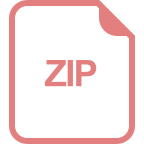
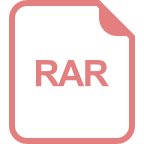
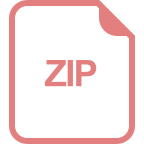


