springboot 跨库分页
时间: 2023-09-26 20:11:30 浏览: 40
在 Spring Boot 中,如果需要进行跨库分页,可以使用 MyBatis 分页插件 PageHelper。
首先,在 pom.xml 文件中添加以下依赖:
```xml
<dependency>
<groupId>com.github.pagehelper</groupId>
<artifactId>pagehelper-spring-boot-starter</artifactId>
<version>1.2.13</version>
</dependency>
```
然后在需要进行分页的方法上添加 `@com.github.pagehelper.PageHelper` 注解,并在方法参数中传入 `PageInfo` 对象,如下所示:
```java
@Service
public class MyService {
@Autowired
private MyMapper myMapper;
@com.github.pagehelper.PageHelper
public List<MyEntity> findMyEntities(PageInfo pageInfo) {
PageHelper.startPage(pageInfo.getPageNum(), pageInfo.getPageSize());
List<MyEntity> myEntities = myMapper.findMyEntities();
return myEntities;
}
}
```
其中,`PageInfo` 是一个自定义的分页信息对象,包含了当前页码和每页显示数量等信息。
在 MyBatis 的 Mapper 接口中,需要使用 `@Mapper` 注解,并在查询方法的参数中添加 `@Param` 注解,如下所示:
```java
@Mapper
public interface MyMapper {
List<MyEntity> findMyEntities();
}
```
最后,在控制器中获取分页数据并返回给前端,如下所示:
```java
@RestController
@RequestMapping("/my")
public class MyController {
@Autowired
private MyService myService;
@GetMapping("/entities")
public PageResult<MyEntity> findMyEntities(PageInfo pageInfo) {
List<MyEntity> myEntities = myService.findMyEntities(pageInfo);
PageResult<MyEntity> pageResult = new PageResult<>(myEntities);
return pageResult;
}
}
```
其中,`PageResult` 是一个自定义的分页结果对象,包含了当前页码、每页显示数量、总记录数和分页数据等信息。
相关推荐
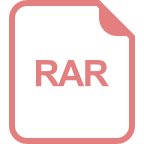
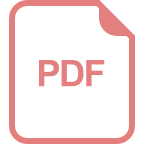














