springboot整合redis配置
时间: 2023-09-14 09:05:55 浏览: 135
### 回答1:
Spring Boot 集成 Redis 配置的方法如下:
1. 在项目的 pom.xml 文件中添加 Redis 依赖:
```
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-redis</artifactId>
</dependency>
```
2. 配置 Redis 连接,在 application.properties 文件中添加如下内容:
```
spring.redis.host=your_redis_host
spring.redis.port=your_redis_port
```
3. 创建 Redis 连接工厂类,在启动类中添加如下代码:
```
@Autowired
private RedisConnectionFactory redisConnectionFactory;
```
4. 在需要使用 Redis 的类中注入 RedisTemplate 对象:
```
@Autowired
private RedisTemplate<String, Object> redisTemplate;
```
以上就是 Spring Boot 集成 Redis 的基本步骤。如果需要更详细的说明,请告诉我您需要了解的细节。
### 回答2:
要将Spring Boot与Redis整合,需要进行以下配置:
1. 在pom.xml文件中添加Redis和Spring Boot的依赖:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-redis</artifactId>
</dependency>
```
2. 在application.properties或application.yml文件中配置Redis的连接信息,包括主机、端口、密码等:
```properties
spring.redis.host=127.0.0.1
spring.redis.port=6379
spring.redis.password=yourpassword
```
3. 创建一个Redis配置类,用于配置Redis连接工厂和Redis模板:
```java
@Configuration
@EnableCaching
public class RedisConfig {
@Bean
public RedisConnectionFactory redisConnectionFactory() {
RedisStandaloneConfiguration configuration = new RedisStandaloneConfiguration();
configuration.setHostName("127.0.0.1");
configuration.setPort(6379);
configuration.setPassword("yourpassword");
return new LettuceConnectionFactory(configuration);
}
@Bean
public RedisTemplate<String, Object> redisTemplate(RedisConnectionFactory factory) {
RedisTemplate<String, Object> template = new RedisTemplate<>();
template.setConnectionFactory(factory);
template.setKeySerializer(new StringRedisSerializer());
template.setValueSerializer(new GenericJackson2JsonRedisSerializer());
return template;
}
}
```
4. 在需要使用Redis的类中通过@Autowired注解获取Redis模板,即可进行Redis操作:
```java
@Autowired
private RedisTemplate<String, Object> redisTemplate;
```
以上就是整合Spring Boot与Redis的配置过程,通过配置Redis连接信息和创建Redis配置类,即可方便地在Spring Boot中使用Redis进行缓存等操作。
### 回答3:
Spring Boot整合Redis的配置主要包括以下几个步骤:
1. 在pom.xml文件中添加Spring Boot和Redis的依赖库,例如:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-redis</artifactId>
</dependency>
```
2. 在application.properties或application.yml配置文件中添加Redis的连接信息,例如:
```properties
spring.redis.host=127.0.0.1
spring.redis.port=6379
spring.redis.password=
```
在这里,我们配置了Redis的主机地址、端口和密码,根据具体情况进行修改。
3. 创建一个RedisTemplate的Bean,并设置相关属性,例如:
```java
@Configuration
public class RedisConfig {
@Bean
public RedisTemplate<String, Object> redisTemplate(RedisConnectionFactory redisConnectionFactory) {
RedisTemplate<String, Object> redisTemplate = new RedisTemplate<>();
redisTemplate.setConnectionFactory(redisConnectionFactory);
// 设置key和value的序列化器
redisTemplate.setKeySerializer(new StringRedisSerializer());
redisTemplate.setValueSerializer(new GenericJackson2JsonRedisSerializer());
return redisTemplate;
}
}
```
在这里,我们使用的是默认的RedisConnectionFactory,同时设置了key和value的序列化器,以便在存储和获取数据时进行对象的序列化和反序列化。
4. 在需要使用Redis的地方,通过@Autowired注解注入redisTemplate,并使用其API进行操作,例如:
```java
@Autowired
private RedisTemplate<String, Object> redisTemplate;
public void setValue(String key, Object value) {
redisTemplate.opsForValue().set(key, value);
}
public Object getValue(String key) {
return redisTemplate.opsForValue().get(key);
}
```
在这里,我们演示了如何设置和获取Redis中的值。
通过以上步骤,就可以在Spring Boot中成功整合Redis,并完成基本的配置。有了Redis的支持,我们可以方便地实现缓存、分布式锁、消息队列等功能。
阅读全文
相关推荐
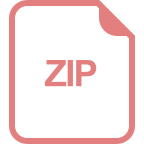












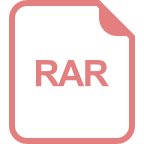
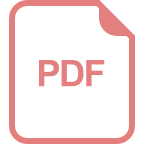