你是编程专家,两块esp8266开发板,sta和ap连接,第一块ESP8266开发板上按键控制第二块ESP8266开发板上15号引脚的LED灯
时间: 2024-05-03 22:16:41 浏览: 11
好的,这个问题需要通过编写代码来实现。首先,需要在第一个ESP8266开发板上编写代码,读取按键状态并将其发送到第二个ESP8266开发板。在第二个ESP8266开发板上,需要编写代码来接收来自第一个开发板的按键状态,并控制15号引脚的LED灯。
以下是第一个ESP8266开发板上的示例代码,用于读取按键状态并将其发送到第二个ESP8266开发板:
```c
#include <ESP8266WiFi.h>
#include <WiFiClient.h>
#include <ESP8266WebServer.h>
const char* ssid = "YOUR_SSID";
const char* password = "YOUR_PASSWORD";
const char* host = "192.168.1.100"; // IP address of the second ESP8266 board
const int port = 80;
int buttonPin = D1; // the pin number of the button
int buttonState = HIGH; // the current state of the button
int lastButtonState = HIGH; // the previous state of the button
WiFiClient client;
ESP8266WebServer server(80);
void setup() {
Serial.begin(115200);
pinMode(buttonPin, INPUT_PULLUP);
WiFi.begin(ssid, password);
while (WiFi.status() != WL_CONNECTED) {
delay(1000);
Serial.println("Connecting to WiFi...");
}
Serial.println("Connected to WiFi");
}
void loop() {
buttonState = digitalRead(buttonPin);
if (buttonState != lastButtonState) {
lastButtonState = buttonState;
if (buttonState == LOW) {
Serial.println("Button pressed");
if (client.connect(host, port)) {
client.println("GET /buttonPressed HTTP/1.1");
client.println("Host: " + String(host));
client.println("Connection: close");
client.println();
delay(100);
client.stop();
} else {
Serial.println("Connection failed");
}
}
}
}
```
在第二个ESP8266开发板上,需要编写代码来接收来自第一个开发板的按键状态,并控制15号引脚的LED灯。以下是示例代码:
```c
#include <ESP8266WiFi.h>
#include <WiFiClient.h>
#include <ESP8266WebServer.h>
const char* ssid = "YOUR_SSID";
const char* password = "YOUR_PASSWORD";
const int port = 80;
int ledPin = 15; // the pin number of the LED
WiFiServer server(80);
ESP8266WebServer webServer(80);
void setup() {
Serial.begin(115200);
pinMode(ledPin, OUTPUT);
WiFi.softAP(ssid, password);
server.begin();
Serial.println("Access point started");
}
void loop() {
WiFiClient client = server.available();
if (client) {
Serial.println("New client");
while (client.connected()) {
if (client.available()) {
String request = client.readStringUntil('\r');
Serial.println(request);
if (request.indexOf("/buttonPressed") != -1) {
digitalWrite(ledPin, HIGH);
delay(1000);
digitalWrite(ledPin, LOW);
}
break;
}
}
client.stop();
Serial.println("Client disconnected");
}
}
```
这两个代码示例可以实现你提出的需求,但需要注意的是,这只是一个简单的示例,实际应用中可能需要更复杂的代码来实现更多的功能、异常处理等。
相关推荐
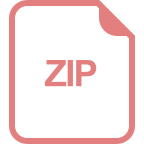
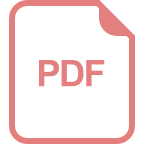
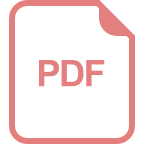














