QTableWidget 表格点击事件
时间: 2024-05-10 13:08:56 浏览: 168
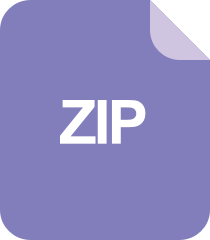
QTableWidget设置表头筛选(含鼠标点击事件过滤)

在 PyQt 中,可以通过 `QTableWidget` 的 `cellClicked` 信号来捕获表格中的单元格点击事件。具体操作步骤如下:
1. 创建一个 `QTableWidget` 控件,并设置列数和行数。
```python
table = QTableWidget()
table.setColumnCount(3)
table.setRowCount(5)
```
2. 为 `cellClicked` 信号连接一个槽函数,该槽函数会在单元格被点击时被调用。
```python
table.cellClicked.connect(self.on_cell_clicked)
```
3. 实现槽函数 `on_cell_clicked`,该函数会接收两个参数,分别是被点击的单元格的行号和列号。
```python
def on_cell_clicked(self, row, column):
# 在这里编写单元格被点击时的操作逻辑
print(f"cell [{row}, {column}] clicked")
```
完整示例代码如下:
```python
from PyQt5.QtWidgets import QApplication, QTableWidget, QWidget, QVBoxLayout
import sys
class Example(QWidget):
def __init__(self):
super().__init__()
self.init_ui()
def init_ui(self):
# 创建表格并设置列数和行数
table = QTableWidget()
table.setColumnCount(3)
table.setRowCount(5)
# 为 cellClicked 信号连接一个槽函数
table.cellClicked.connect(self.on_cell_clicked)
# 将表格添加到窗口中
vbox = QVBoxLayout()
vbox.addWidget(table)
self.setLayout(vbox)
# 设置窗口大小,并显示窗口
self.setGeometry(300, 300, 250, 150)
self.setWindowTitle('QTableWidget')
self.show()
def on_cell_clicked(self, row, column):
# 在这里编写单元格被点击时的操作逻辑
print(f"cell [{row}, {column}] clicked")
if __name__ == '__main__':
app = QApplication(sys.argv)
ex = Example()
sys.exit(app.exec_())
```
运行代码后,单击表格中的任意一个单元格,就会在控制台输出该单元格的行号和列号。
阅读全文
相关推荐
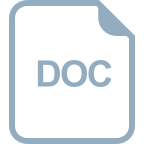
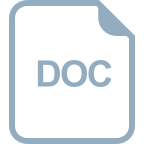
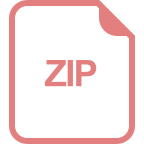
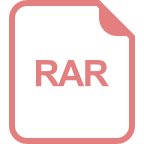






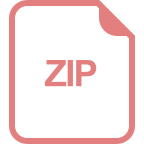
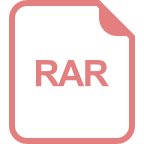
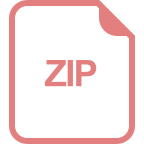
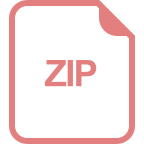
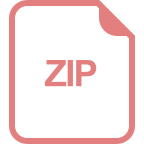


