java遍历entrySet怎么添加元素
时间: 2023-11-22 10:56:57 浏览: 163
遍历 `entrySet` 是用来遍历 Map 类型的数据结构的,它返回一个包含键值对 `Entry` 的 Set 集合。如果要向 Map 中添加元素,可以通过 `put` 方法实现。
以下是一个示例代码:
```java
Map<String, Integer> map = new HashMap<>();
map.put("A", 1);
map.put("B", 2);
for (Map.Entry<String, Integer> entry : map.entrySet()) {
String key = entry.getKey();
Integer value = entry.getValue();
System.out.println(key + " : " + value);
}
// 添加元素
map.put("C", 3);
```
在代码中,我们通过 `entrySet` 方法获取 Map 的键值对集合,然后使用增强型 for 循环遍历集合中的每一个元素。在遍历过程中,我们可以通过 `getKey` 和 `getValue` 方法获取键和值。最后,我们通过 `put` 方法向 Map 中添加元素。
相关问题
java 遍历集合 entrySet()
在Java中,使用entrySet()方法可以遍历集合中的键值对。该方法将集合中的每个元素都转换成一个Entry对象,该对象包含键和值,然后将所有Entry对象存储在Set集合中返回。以下是一个示例代码:
```
Map<String, Integer> map = new HashMap<>();
map.put("a", 1);
map.put("b", 2);
map.put("c", 3);
for (Map.Entry<String, Integer> entry : map.entrySet()) {
System.out.println("Key: " + entry.getKey() + ", Value: " + entry.getValue());
}
```
输出结果为:
```
Key: a, Value: 1
Key: b, Value: 2
Key: c, Value: 3
```
在上述示例中,我们使用了entrySet()方法将map集合转换成Set集合,并使用for循环遍历了该Set集合中的每个Entry对象。在循环中,我们使用entry对象的getKey()和getValue()方法获取了键和值,并将它们打印出来。
java中map遍历每个元素
在Java中,可以使用`Map`的`entrySet()`方法获取所有键值对的集合,然后遍历这个集合来访问每个键值对。具体可以参考以下示例代码:
```java
import java.util.HashMap;
import java.util.Map;
public class Main {
public static void main(String[] args) {
Map<String, Integer> myMap = new HashMap<>();
myMap.put("apple", 3);
myMap.put("banana", 2);
myMap.put("orange", 5);
// 遍历Map中的所有键值对
for (Map.Entry<String, Integer> entry : myMap.entrySet()) {
System.out.println(entry.getKey() + " => " + entry.getValue());
}
}
}
```
上述代码中,通过`myMap.entrySet()`方法获取所有键值对的集合,然后使用for-each循环遍历这个集合。在循环体中,通过`entry.getKey()`和`entry.getValue()`方法访问键值对的键和值。
阅读全文
相关推荐
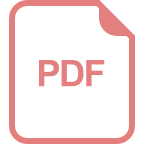
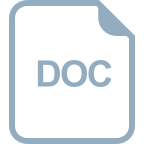
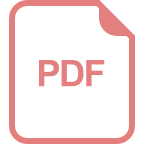
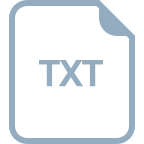
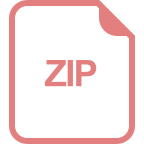
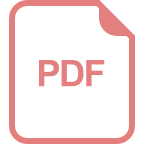
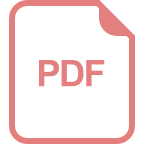
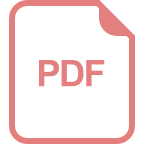
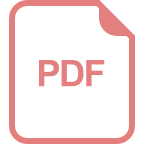





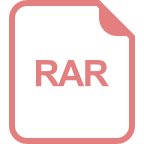