使用c语言完成可以动态修改的配置文件,文件格式如下: pid=10,name=service1,status=1,config=10 pid=11,name=service2,status=1,config=11
时间: 2023-08-18 22:07:55 浏览: 149
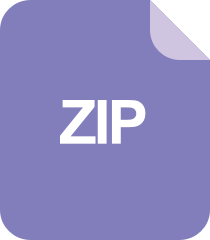
配置文件V2.0版本,主要使用C语言实现
好的,这个任务可以使用C语言中的文件操作和字符串处理来完成。具体的步骤如下:
1. 打开配置文件,使用fopen函数打开文件,打开模式为读写模式("r+")。
2. 读取配置文件内容,使用fgets函数按行读取文件内容,并使用字符串处理函数(如strtok、strchr等)对每一行内容进行解析。将解析后的结果存储在一个结构体数组中。
3. 根据用户输入进行修改,从命令行或其他输入方式读取用户的修改请求,解析后根据pid或其他字段匹配到相应的结构体,对结构体中的相应字段进行修改。
4. 将修改后的结果写入文件,使用fseek函数将文件指针移动到修改前对应的位置,使用fprintf函数将修改后的内容写入文件。
5. 关闭文件,使用fclose函数关闭文件。
下面是一个简单的示例代码,仅供参考:
```c
#include <stdio.h>
#include <string.h>
#define MAX_LINE_LEN 1024
#define MAX_RECORDS 100
struct Record {
int pid;
char name[256];
int status;
int config;
};
int main() {
char filename[] = "config.txt";
FILE *fp = fopen(filename, "r+");
if (fp == NULL) {
printf("Failed to open file %s\n", filename);
return 1;
}
struct Record records[MAX_RECORDS];
int num_records = 0;
char line[MAX_LINE_LEN];
while (fgets(line, MAX_LINE_LEN, fp) != NULL) {
char *token = strtok(line, ",");
struct Record record;
while (token != NULL) {
char *key = strtok(token, "=");
char *value = strtok(NULL, "=");
if (strcmp(key, "pid") == 0) {
record.pid = atoi(value);
} else if (strcmp(key, "name") == 0) {
strcpy(record.name, value);
} else if (strcmp(key, "status") == 0) {
record.status = atoi(value);
} else if (strcmp(key, "config") == 0) {
record.config = atoi(value);
}
token = strtok(NULL, ",");
}
records[num_records++] = record;
}
int pid_to_modify = 10;
int new_status = 0;
for (int i = 0; i < num_records; i++) {
if (records[i].pid == pid_to_modify) {
records[i].status = new_status;
break; // assume pid is unique
}
}
rewind(fp);
for (int i = 0; i < num_records; i++) {
fprintf(fp, "pid=%d,name=%s,status=%d,config=%d\n",
records[i].pid, records[i].name, records[i].status, records[i].config);
}
fclose(fp);
return 0;
}
```
阅读全文
相关推荐
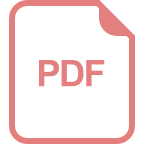
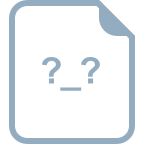
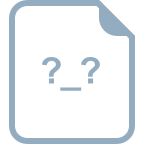
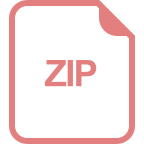
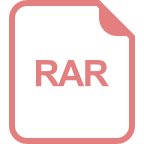
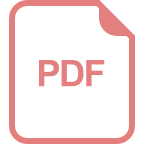
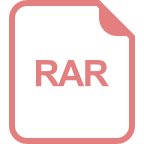
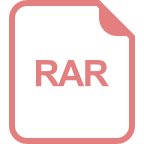
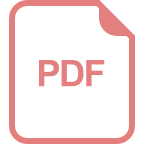
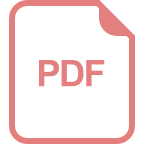
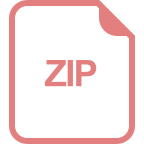
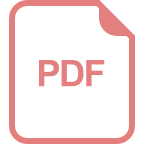
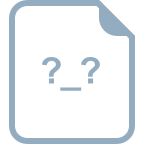
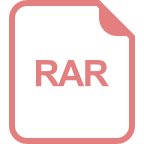