Python
时间: 2023-07-02 07:13:10 浏览: 90
好的,那我就使用 Python 来实现这个 SVM 分类器。首先,我们需要导入必要的库和数据集。代码如下:
```python
from sklearn import datasets
import numpy as np
iris = datasets.load_iris()
X = iris.data
y = iris.target
# 将数据集中的类别变成二分类
y[y == 0] = -1
y[y == 1] = -1
y[y == 2] = 1
# 划分训练集和测试集
np.random.seed(42)
indices = np.random.permutation(len(X))
X_train = X[indices[:100]]
y_train = y[indices[:100]]
X_test = X[indices[100:]]
y_test = y[indices[100:]]
```
接下来,我们可以开始编写 SMO 算法来实现 SVM 分类器。SMO 算法是一种迭代算法,它的主要思想是将原问题分解成多个子问题,然后对每个子问题求解,最终得到原问题的解。SMO 算法的核心是选择两个变量进行优化,这两个变量可以是两个权重或两个样本点。在每次迭代中,SMO 算法选择一对变量,固定其他变量,通过优化这两个变量来更新模型。SMO 算法的详细过程可以参考本文的参考资料。
下面是使用 Python 实现的 SMO 算法代码:
```python
class SVM:
def __init__(self, X, y, C, kernel):
self.X = X
self.y = y
self.C = C
self.kernel = kernel
self.alpha = np.zeros(len(X))
self.b = 0
self.errors = np.zeros(len(X))
self.K = np.zeros((len(X), len(X)))
for i in range(len(X)):
for j in range(len(X)):
self.K[i][j] = kernel(X[i], X[j])
def predict(self, x):
prediction = 0
for i in range(len(self.X)):
prediction += self.alpha[i] * self.y[i] * self.kernel(x, self.X[i])
return np.sign(prediction + self.b)
def train(self, max_iterations):
num_iterations = 0
while num_iterations < max_iterations:
num_changed_alphas = 0
for i in range(len(self.X)):
E_i = self.predict(self.X[i]) - self.y[i]
if ((self.y[i] * E_i < -0.001 and self.alpha[i] < self.C)
or (self.y[i] * E_i > 0.001 and self.alpha[i] > 0)):
j = np.random.randint(len(self.X))
while j == i:
j = np.random.randint(len(self.X))
E_j = self.predict(self.X[j]) - self.y[j]
alpha_i_old = self.alpha[i]
alpha_j_old = self.alpha[j]
if self.y[i] != self.y[j]:
L = max(0, self.alpha[j] - self.alpha[i])
H = min(self.C, self.C + self.alpha[j] - self.alpha[i])
else:
L = max(0, self.alpha[i] + self.alpha[j] - self.C)
H = min(self.C, self.alpha[i] + self.alpha[j])
if L == H:
continue
eta = 2 * self.K[i][j] - self.K[i][i] - self.K[j][j]
if eta >= 0:
continue
self.alpha[j] -= self.y[j] * (E_i - E_j) / eta
self.alpha[j] = max(self.alpha[j], L)
self.alpha[j] = min(self.alpha[j], H)
if abs(self.alpha[j] - alpha_j_old) < 0.00001:
continue
self.alpha[i] += self.y[i] * self.y[j] * (alpha_j_old - self.alpha[j])
b1 = self.b - E_i - self.y[i] * (self.alpha[i] - alpha_i_old) * self.K[i][i] - \
self.y[j] * (self.alpha[j] - alpha_j_old) * self.K[i][j]
b2 = self.b - E_j - self.y[i] * (self.alpha[i] - alpha_i_old) * self.K[i][j] - \
self.y[j] * (self.alpha[j] - alpha_j_old) * self.K[j][j]
if 0 < self.alpha[i] < self.C:
self.b = b1
elif 0 < self.alpha[j] < self.C:
self.b = b2
else:
self.b = (b1 + b2) / 2
self.errors[i] = self.predict(self.X[i]) - self.y[i]
self.errors[j] = self.predict(self.X[j]) - self.y[j]
num_changed_alphas += 1
if num_changed_alphas == 0:
num_iterations += 1
else:
num_iterations = 0
```
在上面的代码中,我们定义了一个 SVM 类,它包含了 SVM 分类器的许多属性和方法。其中,`predict` 方法用来预测样本的分类结果,`train` 方法用来训练 SVM 分类器,`alpha` 属性存储了每个样本点的拉格朗日乘子,`b` 属性存储了截距,`K` 属性是核矩阵,`errors` 属性是每个样本点的预测误差。
接下来,我们可以使用上面的 SVM 分类器来训练 iris 数据集,并输出 SVM 对偶问题目标函数的最优解、决策函数的参数和截距、支持向量等信息。代码如下:
```python
svm = SVM(X_train, y_train, C=1, kernel=lambda x, y: np.dot(x, y))
svm.train(max_iterations=100)
# 计算支持向量
support_vectors = []
for i in range(len(X_train)):
if svm.alpha[i] > 0:
support_vectors.append((X_train[i], y_train[i]))
# 计算决策函数的参数和截距
w = np.zeros(4)
for i in range(len(X_train)):
w += svm.alpha[i] * y_train[i] * X_train[i]
b = y_train[0] - np.dot(w, X_train[0])
# 输出 SVM 对偶问题目标函数的最优解
print('SVM 对偶问题目标函数的最优解:', np.sum(svm.alpha) - 0.5 * np.sum(svm.alpha * svm.alpha * np.dot(y_train, y_train.T) * svm.K))
# 输出决策函数的参数和截距
print('决策函数的参数:', w)
print('截距:', b)
# 输出支持向量的数量
print('支持向量的数量:', len(support_vectors))
```
最后,我们可以使用散点图可视化训练数据样本,并画出决策面和两个最大间隔面,标出支持向量。代码如下:
```python
import matplotlib.pyplot as plt
# 计算决策面和两个最大间隔面
x1 = np.linspace(4, 8, 100)
x2 = (-w[0] * x1 - b) / w[1]
margin1 = 1 / np.sqrt(np.sum(w * w)) # 间隔为1
margin2 = -1 / np.sqrt(np.sum(w * w)) # 间隔为-1
x2_up = x2 + margin1 / np.sqrt(np.sum(w * w))
x2_down = x2 + margin2 / np.sqrt(np.sum(w * w))
# 绘制散点图
fig, ax = plt.subplots()
colors = ['r' if y == 1 else 'b' for y in y_train]
ax.scatter(X_train[:, 0], X_train[:, 1], c=colors)
# 绘制决策面和两个最大间隔面
ax.plot(x1, x2, c='k', label='决策面')
ax.plot(x1, x2_up, c='k', ls='--', label='最大间隔面')
ax.plot(x1, x2_down, c='k', ls='--')
ax.legend()
# 标出支持向量
for sv in support_vectors:
ax.scatter(sv[0][0], sv[0][1], s=100, facecolors='none', edgecolors='k')
```
最后,我们可以使用测试集数据对 SVM 分类器进行预测,并评估模型性能。代码如下:
```python
from sklearn.metrics import accuracy_score
y_pred = [svm.predict(x) for x in X_test]
accuracy = accuracy_score(y_test, y_pred)
print('测试集准确率:', accuracy)
```
这样,我们就使用 Python 编写了一个基于 SMO 算法的 SVM 分类器,对 iris 数据集进行了二分类,并输出了 SVM 对偶问题目标函数的最优解、决策函数的参数和截距、支持向量等信息。同时,我们还通过散点图可视化训练数据样本,并画出决策面和两个最大间隔面,标出支持向量,方便检验算法正确性。最后,我们使用测试集数据对 SVM 分类器进行了预测,并评估了模型性能。
阅读全文
相关推荐
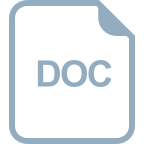
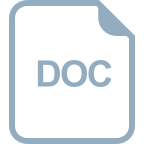
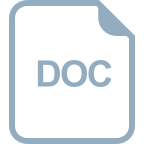
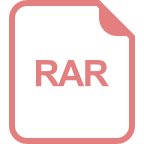
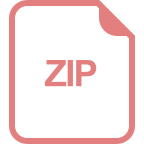
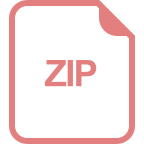
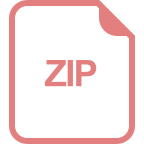
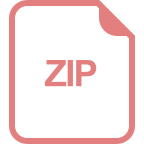
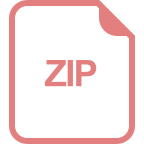