基于 AES 算法的 CTR 模式演示程序用c语言
时间: 2024-05-07 16:20:57 浏览: 215
以下是一个基于AES算法的CTR模式的演示程序,使用了C语言和OpenSSL库:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <openssl/aes.h>
#define BLOCK_SIZE 16 // AES-128 block size
// Encrypt or decrypt data using AES-128-CTR mode
void aes_ctr_cipher(const unsigned char *key, const unsigned char *iv, const unsigned char *input, unsigned char *output, size_t length) {
AES_KEY aes_key;
AES_set_encrypt_key(key, BLOCK_SIZE * 8, &aes_key); // Use AES-128
unsigned char ecount_buf[BLOCK_SIZE] = {0}; // Counter block
unsigned int num = 0; // Bit counter
AES_ctr128_encrypt(input, output, length, &aes_key, iv, ecount_buf, &num);
}
int main() {
unsigned char key[BLOCK_SIZE] = "1234567890123456"; // 16-byte key
unsigned char iv[BLOCK_SIZE] = "abcdefghijklmnop"; // 16-byte initialization vector
unsigned char input[] = "Hello, world!"; // Plaintext message
size_t input_len = strlen((char*)input);
unsigned char output[input_len];
aes_ctr_cipher(key, iv, input, output, input_len);
printf("Encrypted message: %s\n", output);
aes_ctr_cipher(key, iv, output, input, input_len); // Decrypt message
printf("Decrypted message: %s\n", input);
return 0;
}
```
该程序使用了OpenSSL库,需要在编译时链接该库,例如:
```
gcc -o aes_ctr_cipher aes_ctr_cipher.c -lcrypto
```
其中,`aes_ctr_cipher`是生成的可执行文件名。运行程序后,将输出加密后的消息和解密后的消息。
阅读全文
相关推荐



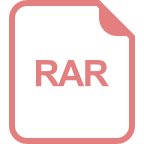










