.NET core手机验证码 免费教程
时间: 2023-08-31 20:27:21 浏览: 56
### 回答1:
以下是.NET Core实现手机验证码的免费教程:
1. 首先,你需要在你的.NET Core项目中安装 `Twilio` 包,它是一个非常好用的第三方库,可以帮助你发送短信。
2. 接下来,你需要在 `Startup.cs` 文件中的 `ConfigureServices` 方法中添加以下代码:
```csharp
services.Configure<TwilioSettings>(Configuration.GetSection("Twilio"));
services.AddSingleton<ITwilioRestClient>(s => new TwilioRestClient(
s.GetRequiredService<IOptions<TwilioSettings>>().Value.AccountSid,
s.GetRequiredService<IOptions<TwilioSettings>>().Value.AuthToken));
services.AddTransient<ISmsService, SmsService>();
```
3. 在 `appsettings.json` 文件中添加以下代码:
```json
"Twilio": {
"AccountSid": "你的Twilio账户SID",
"AuthToken": "你的Twilio账户AuthToken",
"PhoneNumber": "你的Twilio手机号码"
}
```
4. 创建 `TwilioSettings` 类:
```csharp
public class TwilioSettings
{
public string AccountSid { get; set; }
public string AuthToken { get; set; }
public string PhoneNumber { get; set; }
}
```
5. 创建 `ISmsService` 接口:
```csharp
public interface ISmsService
{
Task<bool> SendSmsAsync(string phoneNumber, string message);
}
```
6. 创建 `SmsService` 类:
```csharp
public class SmsService : ISmsService
{
private readonly ITwilioRestClient _twilioRestClient;
private readonly TwilioSettings _twilioSettings;
public SmsService(ITwilioRestClient twilioRestClient, IOptions<TwilioSettings> twilioSettings)
{
_twilioRestClient = twilioRestClient;
_twilioSettings = twilioSettings.Value;
}
public async Task<bool> SendSmsAsync(string phoneNumber, string message)
{
try
{
var result = await MessageResource.CreateAsync(
body: message,
from: new Twilio.Types.PhoneNumber(_twilioSettings.PhoneNumber),
to: new Twilio.Types.PhoneNumber(phoneNumber),
client: _twilioRestClient);
return result != null;
}
catch (Exception ex)
{
// Handle exception here
return false;
}
}
}
```
7. 最后,在需要发送验证码的地方,注入 `ISmsService` 接口,调用 `SendSmsAsync` 方法即可:
```csharp
public class VerificationController : ControllerBase
{
private readonly ISmsService _smsService;
public VerificationController(ISmsService smsService)
{
_smsService = smsService;
}
[HttpPost]
public async Task<IActionResult> SendVerificationCode(string phoneNumber)
{
// Generate verification code here
var verificationCode = "123456";
var message = $"Your verification code is: {verificationCode}";
var result = await _smsService.SendSmsAsync(phoneNumber, message);
if (result)
{
return Ok();
}
else
{
return BadRequest();
}
}
}
```
以上就是.NET Core实现手机验证码的免费教程了,希望对你有所帮助!
### 回答2:
.NET Core 是微软推出的跨平台开发框架,它具有高性能、可扩展性和跨平台的特性,广受开发者的欢迎。当我们在开发手机App时,往往需要用到手机验证码的功能,以提供一种用户身份验证的方式。
在.NET Core中,我们可以通过短信服务提供商来实现手机验证码功能。下面是一种免费的教程,演示如何通过.NET Core发送手机验证码:
1. 首先,选择一个短信服务提供商,比如阿里云、腾讯云等。这些提供商通常都有.NET Core的SDK,可以在官方文档中找到教程和示例代码。
2. 在.NET Core项目中,通过NuGet安装短信服务提供商的SDK。可以通过在Visual Studio的NuGet包管理器中搜索相关关键字,找到适合的SDK并安装。
3. 在项目中引入短信服务提供商的命名空间,并创建一个短信服务客户端对象。根据SDK的文档,可以找到创建客户端的示例代码。
4. 设置短信服务提供商的账号信息,比如Access Key、Secret Key等。这些信息可以在短信服务提供商的控制台中获取,用于验证开发者的身份。
5. 实现发送手机验证码的逻辑。根据短信服务提供商的API文档,找到发送短信验证码的接口或方法,并调用它来发送验证码。
6. 在用户注册或登录的业务逻辑中,调用发送手机验证码的逻辑,并将验证码发送到指定的手机号码。可以通过用户输入的手机号码和接收到的验证码来验证用户的身份。
需要注意的是,免费教程中使用的短信服务提供商可能会有一定的限制,比如每天或每月发送短信的次数限制。如果需要更高的发送频率或更多的功能,可能需要购买相应的套餐或服务。
以上是一个大致的教程,具体的实现细节和步骤可能会因为不同的短信服务提供商而有所差异。但通过学习和掌握.NET Core的使用以及相应的短信服务提供商的API,我们可以很容易地实现手机验证码的功能。
相关推荐
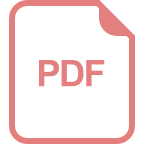
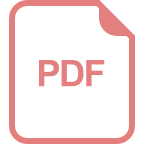














