使用头插法建立一个带头结点的存储学生信息(学号, 姓名)的单链表,并将建立后的学生链表中的信息输出到屏幕上。 具体要求: ① 单链表的建立和单链表的显示分别使用函数实现,学号为0,结束输入。 ② 在main函数中完成初始化工作并调用上述两个函数。
时间: 2024-02-13 22:59:44 浏览: 79
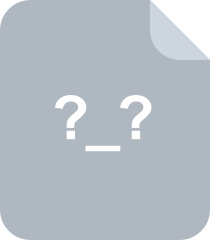
头插法建立单链表
好的,这是一个关于链表的问题,我来和你一步步解答。
首先,我们需要定义一个学生结构体,包含学号和姓名两个成员变量,代码如下:
```c++
struct Student {
int id;
string name;
};
```
接下来,我们需要定义一个链表结点结构体,包含一个学生结构体和一个指向下一个结点的指针,代码如下:
```c++
struct ListNode {
Student student;
ListNode* next;
};
```
注意,这里的指针要使用指向结点的指针,而不是指向学生结构体的指针。
接下来,我们就可以使用头插法来建立链表了。头插法的思路是,每输入一个学生信息,就新建一个链表结点,把它插入到链表头部。
具体实现代码如下:
```c++
ListNode* createList() {
ListNode* head = new ListNode(); // 新建头结点
head->next = nullptr; // 头结点的 next 指针置为 nullptr
Student student; // 存储学生信息
cout << "请输入学生信息(学号为0,结束输入):" << endl;
cin >> student.id;
while (student.id != 0) {
cin >> student.name;
ListNode* node = new ListNode(); // 新建结点
node->student = student; // 把学生信息存入结点中
node->next = head->next; // 把当前结点的 next 指针指向链表头部
head->next = node; // 把当前结点插入到链表头部
cin >> student.id; // 继续输入下一个学生信息
}
return head; // 返回链表头结点
}
```
最后,我们需要一个函数来遍历链表,输出所有学生信息。遍历链表的方法是,从链表头部开始,依次访问每个结点,输出学生信息。
具体实现代码如下:
```c++
void printList(ListNode* head) {
cout << "学号\t姓名" << endl;
ListNode* p = head->next; // 从链表头部开始访问结点
while (p != nullptr) { // 如果当前结点不为空
cout << p->student.id << "\t" << p->student.name << endl; // 输出当前结点的学生信息
p = p->next; // 继续访问下一个结点
}
}
```
最后,我们在 main 函数中调用 createList 函数和 printList 函数即可。完整代码如下:
```c++
#include <iostream>
using namespace std;
struct Student {
int id;
string name;
};
struct ListNode {
Student student;
ListNode* next;
};
ListNode* createList() {
ListNode* head = new ListNode(); // 新建头结点
head->next = nullptr; // 头结点的 next 指针置为 nullptr
Student student; // 存储学生信息
cout << "请输入学生信息(学号为0,结束输入):" << endl;
cin >> student.id;
while (student.id != 0) {
cin >> student.name;
ListNode* node = new ListNode(); // 新建结点
node->student = student; // 把学生信息存入结点中
node->next = head->next; // 把当前结点的 next 指针指向链表头部
head->next = node; // 把当前结点插入到链表头部
cin >> student.id; // 继续输入下一个学生信息
}
return head; // 返回链表头结点
}
void printList(ListNode* head) {
cout << "学号\t姓名" << endl;
ListNode* p = head->next; // 从链表头部开始访问结点
while (p != nullptr) { // 如果当前结点不为空
cout << p->student.id << "\t" << p->student.name << endl; // 输出当前结点的学生信息
p = p->next; // 继续访问下一个结点
}
}
int main() {
ListNode* head = createList(); // 建立链表
printList(head); // 输出链表
return 0;
}
```
如果你在输入学生信息时,输入了如下数据:
```
001 Alice
002 Bob
003 Charlie
004 David
005 Emma
000
```
则程序的输出结果应该为:
```
学号 姓名
005 Emma
004 David
003 Charlie
002 Bob
001 Alice
```
阅读全文
相关推荐






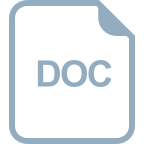
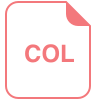

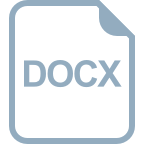
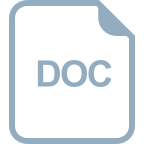
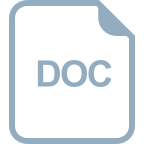
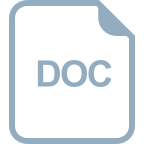
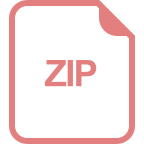
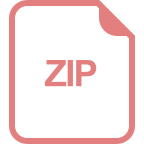
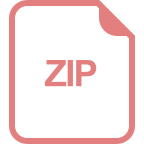