Serializable,具体代码例子
时间: 2023-04-09 15:00:57 浏览: 95
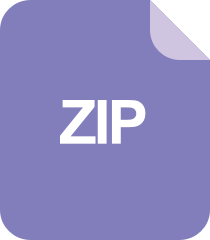
序列化示例代码
Serializable 是 Java 中的一个接口,用于实现对象的序列化和反序列化。下面是一个简单的代码例子:
```
import java.io.*;
public class Person implements Serializable {
private String name;
private int age;
public Person(String name, int age) {
this.name = name;
this.age = age;
}
public void printInfo() {
System.out.println("Name: " + name);
System.out.println("Age: " + age);
}
public static void main(String[] args) {
Person person = new Person("Tom", 20);
try {
FileOutputStream fileOut = new FileOutputStream("person.ser");
ObjectOutputStream out = new ObjectOutputStream(fileOut);
out.writeObject(person);
out.close();
fileOut.close();
System.out.println("Serialized data is saved in person.ser");
} catch (IOException i) {
i.printStackTrace();
}
try {
FileInputStream fileIn = new FileInputStream("person.ser");
ObjectInputStream in = new ObjectInputStream(fileIn);
Person newPerson = (Person) in.readObject();
in.close();
fileIn.close();
System.out.println("Deserialized data:");
newPerson.printInfo();
} catch (IOException i) {
i.printStackTrace();
return;
} catch (ClassNotFoundException c) {
System.out.println("Person class not found");
c.printStackTrace();
return;
}
}
}
```
在这个例子中,我们定义了一个 Person 类,实现了 Serializable 接口。我们将一个 Person 对象序列化到文件中,然后再从文件中反序列化出来,并打印出对象的信息。
阅读全文
相关推荐
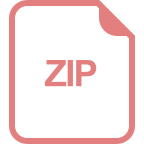
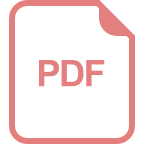
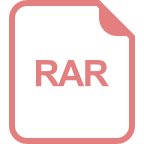
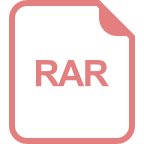
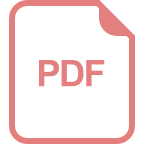
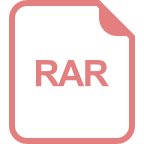
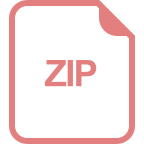
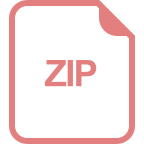
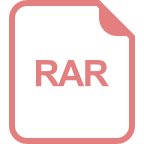
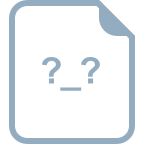
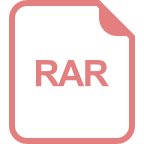
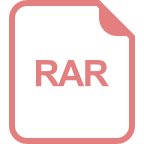
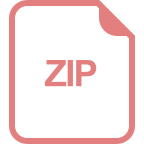
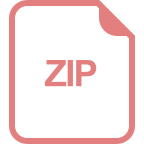
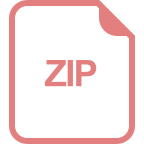
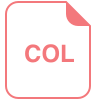
