编程实现朴素贝叶斯分类算法
时间: 2023-08-14 21:29:58 浏览: 98
好的,朴素贝叶斯分类算法是一种基于贝叶斯定理的分类算法。它假设每个特征之间相互独立,并且假设每个特征对分类结果的影响是一样的。
下面是朴素贝叶斯分类算法的实现步骤:
1. 收集数据:采集训练数据,数据应该包括特征和分类结果。
2. 准备数据:将数据转化为适合分类器使用的格式。对于朴素贝叶斯分类器,需要将数据转化为词向量或者数字向量的形式。
3. 分析数据:可以使用可视化工具对数据进行初步分析。
4. 训练算法:计算不同分类下特征的条件概率,即P(feature|class)。
5. 测试算法:使用一部分数据集来测试分类器的准确性。
6. 使用算法:将分类器应用到实际应用中。
下面是 Python 实现朴素贝叶斯分类器的代码:
```python
import numpy as np
class NaiveBayes:
def __init__(self):
self.prior_prob = None
self.cond_prob = None
def train(self, X, y):
self.prior_prob = {}
self.cond_prob = {}
n_samples, n_features = X.shape
classes = np.unique(y)
for c in classes:
X_c = X[y == c]
self.prior_prob[c] = X_c.shape[0] / n_samples
self.cond_prob[c] = {}
for i in range(n_features):
self.cond_prob[c][i] = {}
for value in np.unique(X[:, i]):
self.cond_prob[c][i][value] = (X_c[:, i] == value).sum() / X_c.shape[0]
def predict(self, X):
classes = list(self.prior_prob.keys())
n_samples, n_features = X.shape
prob = np.zeros((n_samples, len(classes)))
for i in range(len(classes)):
c = classes[i]
prob_c = self.prior_prob[c]
for j in range(n_features):
prob_c *= self.cond_prob[c][j][X[i, j]]
prob[i, i] = prob_c
return classes[np.argmax(prob, axis=1)]
```
这个实现中,train 函数用于训练分类器,predict 函数用于预测分类结果。其中,prior_prob 存储了每个类别的先验概率,cond_prob 存储了每个类别下,每个特征的条件概率。在预测时,首先计算每个类别的概率,再算出每个样本的条件概率,最后返回概率最大的类别作为分类结果。
阅读全文
相关推荐
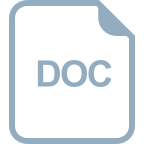
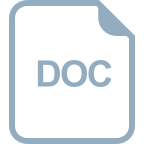
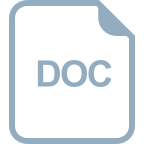
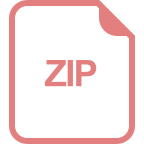
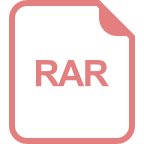
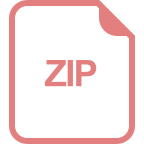
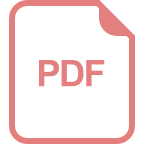
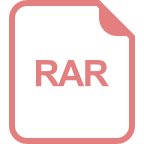
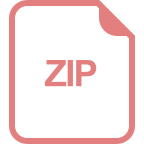
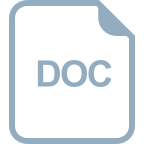
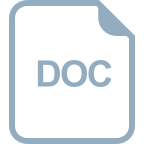
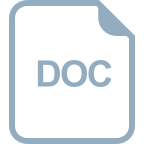
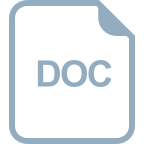
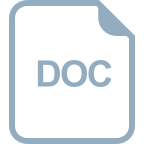



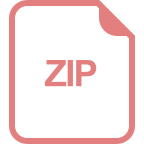
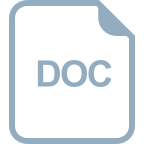