冒泡排序 实现对一个无序的链表排序 java代码
时间: 2023-06-04 07:04:34 浏览: 58
以下是Java实现冒泡排序的代码:
```
public class LinkedListBubbleSort {
public static void bubbleSortLinkedList(Node head) {
if (head == null) {
return;
}
boolean swapped = true;
while (swapped) {
swapped = false;
Node previous = null, current = head;
while (current.next != null) {
if (current.data > current.next.data) {
// swap two adjacent nodes
Node nextNode = current.next;
current.next = nextNode.next;
nextNode.next = current;
if (previous != null) {
previous.next = nextNode;
} else {
head = nextNode;
}
previous = nextNode;
swapped = true;
} else {
previous = current;
current = current.next;
}
}
}
}
public static void main(String[] args) {
Node node1 = new Node(5);
Node node2 = new Node(3);
Node node3 = new Node(8);
Node node4 = new Node(1);
Node node5 = new Node(2);
Node node6 = new Node(5);
node1.next = node2;
node2.next = node3;
node3.next = node4;
node4.next = node5;
node5.next = node6;
node6.next = null;
bubbleSortLinkedList(node1);
Node current = node1;
while (current != null) {
System.out.print(current.data + " ");
current = current.next;
}
}
private static class Node {
int data;
Node next;
Node(int data) {
this.data = data;
}
}
}
```
上面的代码使用冒泡排序算法对一个无序的单向链表进行排序,思路是每次遍历链表,将相邻两个元素中较大的那个交换到后面,直到没有需要交换的元素为止。时间复杂度为O(n^2)。
相关推荐
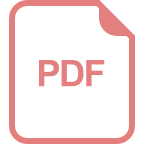














