HTML5绘制一个3D立方体,HTML5/Canvas交互式3D立方体
时间: 2024-01-15 16:02:37 浏览: 35
好的,下面是HTML5/Canvas交互式3D立方体的代码,你可以直接复制粘贴到HTML文件中进行实验:
```html
<!DOCTYPE html>
<html>
<head>
<style>
canvas {
border: 1px solid black;
}
</style>
</head>
<body>
<canvas id="canvas" width="400" height="400"></canvas>
<script>
var canvas = document.getElementById("canvas");
var ctx = canvas.getContext("2d");
// 定义立方体的六个面
var cube = [
{ x: -50, y: -50, z: -50 },
{ x: -50, y: 50, z: -50 },
{ x: 50, y: 50, z: -50 },
{ x: 50, y: -50, z: -50 },
{ x: -50, y: -50, z: 50 },
{ x: -50, y: 50, z: 50 },
{ x: 50, y: 50, z: 50 },
{ x: 50, y: -50, z: 50 },
];
// 定义立方体的六个面的连接方式
var connections = [
[0, 1, 2, 3],
[4, 5, 6, 7],
[0, 4, 5, 1],
[1, 5, 6, 2],
[2, 6, 7, 3],
[3, 7, 4, 0],
];
// 定义立方体的初始位置和旋转角度
var position = { x: 200, y: 200, z: 0 };
var rotation = { x: 0, y: 0, z: 0 };
// 绘制立方体
function draw() {
ctx.clearRect(0, 0, canvas.width, canvas.height);
ctx.save();
ctx.translate(position.x, position.y);
ctx.rotate(rotation.z);
ctx.rotate(rotation.y);
ctx.rotate(rotation.x);
ctx.translate(-position.x, -position.y);
for (var i = 0; i < connections.length; i++) {
ctx.beginPath();
for (var j = 0; j < connections[i].length; j++) {
var p = project(cube[connections[i][j]]);
if (j == 0) {
ctx.moveTo(p.x, p.y);
} else {
ctx.lineTo(p.x, p.y);
}
}
ctx.closePath();
ctx.stroke();
}
ctx.restore();
}
// 投影函数,将3D坐标点投影到2D平面上
function project(p) {
var scale = 200 / (200 + p.z + position.z);
return {
x: p.x * scale + position.x,
y: p.y * scale + position.y,
};
}
// 鼠标控制旋转
var mousedown = false;
var mouse = { x: 0, y: 0 };
canvas.addEventListener("mousedown", function (e) {
mousedown = true;
mouse.x = e.clientX;
mouse.y = e.clientY;
});
canvas.addEventListener("mouseup", function () {
mousedown = false;
});
canvas.addEventListener("mousemove", function (e) {
if (mousedown) {
rotation.x += (e.clientY - mouse.y) * 0.01;
rotation.y += (e.clientX - mouse.x) * 0.01;
mouse.x = e.clientX;
mouse.y = e.clientY;
draw();
}
});
// 渲染循环
function loop() {
requestAnimationFrame(loop);
draw();
}
loop();
</script>
</body>
</html>
```
这个代码将会在canvas标签中绘制一个3D立方体,并且根据鼠标的控制进行旋转。
相关推荐
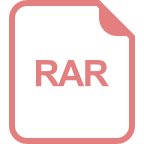
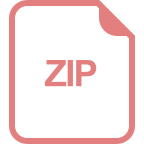














