基于MVVM的中英文单词计数器的设计与实现
时间: 2023-08-05 17:03:41 浏览: 44
MVVM是一种软件架构模式,它将用户界面(UI)、应用程序逻辑和数据模型分为三个不同的组件来进行开发和管理。在基于MVVM的中英文单词计数器的设计与实现中,我们可以按照以下步骤进行:
1. 模型层(M):定义数据模型和数据操作方法,用于存储和操作数据。在这个例子中,我们需要定义一个数据模型用于存储文本数据,以及一个方法用于计算文本中的中英文单词数量。
2. 视图层(V):定义用户界面,用于展示数据和接收用户操作。在这个例子中,我们需要定义一个输入框用于输入文本,一个计数框用于展示计数结果。
3. 视图模型层(VM):连接模型层和视图层,用于处理视图层和模型层之间的数据传递。在这个例子中,我们需要定义一个视图模型用于将视图层和模型层进行绑定,以便将计算结果展示到计数框中。
接下来是具体的实现步骤:
1. 模型层(M):我们可以定义一个名为WordCounter的类来实现模型层。该类中包含一个存储文本数据的字符串变量和一个计算中英文单词数量的方法。方法的实现可以使用正则表达式来匹配中英文单词,例如:
```csharp
public class WordCounter
{
private string text;
public WordCounter(string text)
{
this.text = text;
}
public int CountWords()
{
string pattern = @"([\u4e00-\u9fa5]+)|([a-zA-Z]+)";
Regex regex = new Regex(pattern);
MatchCollection matches = regex.Matches(text);
return matches.Count;
}
}
```
2. 视图层(V):我们可以使用WPF框架来实现视图层。在XAML文件中,我们可以添加一个输入框和一个计数框,代码如下:
```xaml
<Window x:Class="WordCounter.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="Word Counter" Height="200" Width="300">
<Grid>
<StackPanel Margin="10">
<Label Content="Input text:"/>
<TextBox x:Name="InputBox" Margin="0 5 0 10"/>
<Label Content="Word count:"/>
<TextBox x:Name="CountBox" IsReadOnly="True"/>
</StackPanel>
</Grid>
</Window>
```
3. 视图模型层(VM):我们可以定义一个名为WordCounterViewModel的类来实现视图模型层。该类中包含一个处理计数按钮点击事件的方法,在该方法中调用模型层的计数方法,并将结果绑定到计数框中。代码如下:
```csharp
public class WordCounterViewModel : INotifyPropertyChanged
{
private string inputText;
private int wordCount;
public event PropertyChangedEventHandler PropertyChanged;
public string InputText
{
get { return inputText; }
set
{
inputText = value;
OnPropertyChanged("InputText");
}
}
public int WordCount
{
get { return wordCount; }
set
{
wordCount = value;
OnPropertyChanged("WordCount");
}
}
public void CountWords()
{
WordCounter counter = new WordCounter(InputText);
WordCount = counter.CountWords();
}
protected void OnPropertyChanged(string propertyName)
{
PropertyChanged?.Invoke(this, new PropertyChangedEventArgs(propertyName));
}
}
```
在该类中,我们使用了INotifyPropertyChanged接口来实现属性变化通知。当InputText和WordCount属性的值发生变化时,该类会自动触发OnPropertyChanged方法,通知绑定到这些属性的视图层进行更新。
最后,我们需要在代码中实现视图层和视图模型层的绑定。在MainWindow类的构造函数中,我们可以创建一个WordCounterViewModel对象,并将该对象与视图层进行绑定。代码如下:
```csharp
public partial class MainWindow : Window
{
private WordCounterViewModel viewModel;
public MainWindow()
{
InitializeComponent();
viewModel = new WordCounterViewModel();
DataContext = viewModel;
}
private void CountButton_Click(object sender, RoutedEventArgs e)
{
viewModel.CountWords();
}
}
```
在该代码中,我们将视图模型层对象赋值给DataContext属性,从而实现了视图层和视图模型层之间的绑定。当计数按钮被点击时,会触发CountButton_Click事件,该事件会调用视图模型层的CountWords方法,并将计算结果绑定到计数框中。
这样,我们就完成了基于MVVM的中英文单词计数器的设计与实现。
相关推荐
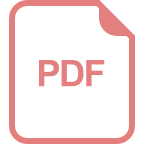
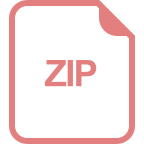
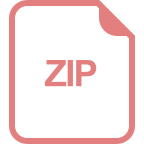
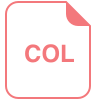













