c++和linux实现遍历路径下所有文件和目录,并输出文件和目录的名称
时间: 2024-03-06 14:50:45 浏览: 38
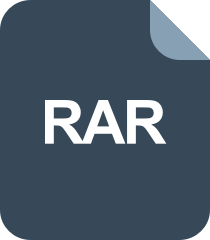
rm.rar_LINUX 遍历文件_linux rm_rm_rm linux_rm2013目录
可以使用 opendir() 和 readdir() 函数来遍历目录下的所有文件和子目录。
以下是一个 C++ 示例代码:
```c++
#include <iostream>
#include <dirent.h>
#include <string>
void list_files(const std::string& path) {
DIR *dir;
struct dirent *ent;
if ((dir = opendir(path.c_str())) != NULL) {
while ((ent = readdir(dir)) != NULL) {
if(ent->d_type == DT_DIR) {
if (std::string(ent->d_name) != "." && std::string(ent->d_name) != "..") {
std::cout << "Directory: " << ent->d_name << std::endl;
std::string sub_path = path + "/" + std::string(ent->d_name);
list_files(sub_path);
}
} else {
std::cout << "File: " << ent->d_name << std::endl;
}
}
closedir(dir);
} else {
std::cerr << "Failed to open directory: " << path << std::endl;
}
}
int main(int argc, char* argv[]) {
if (argc != 2) {
std::cerr << "Usage: " << argv[0] << " <path>" << std::endl;
return 1;
}
std::string path = argv[1];
list_files(path);
return 0;
}
```
在上面的代码中,我们定义了一个递归函数 list_files(),它可以遍历指定路径下的所有文件和子目录。
我们首先使用 opendir() 函数打开指定路径,然后使用 readdir() 函数逐个读取目录项。如果目录项是一个子目录,则递归调用 list_files() 函数来遍历该子目录。否则,该目录项是一个文件,我们就输出它的名称。
在 main() 函数中,我们从命令行参数中获取指定的路径,并调用 list_files() 函数来遍历该路径下的所有文件和子目录。
你可以将上面的示例代码复制到一个 cpp 文件中,然后在命令行中使用 g++ 编译器编译并运行,例如:
```bash
g++ -o list_files list_files.cpp
./list_files /path/to/directory
```
这将输出指定目录下所有文件和子目录的名称。
阅读全文
相关推荐
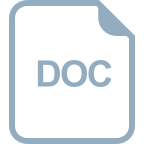
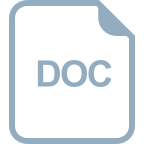


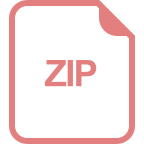
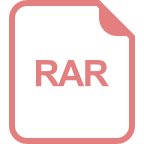



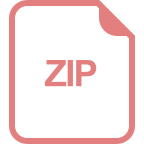




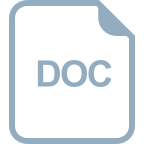
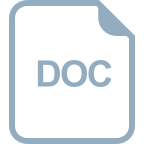
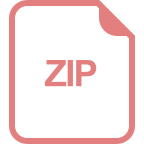
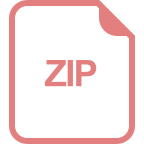
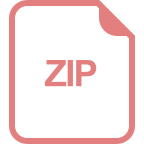