完成四则运算登陆模块(至少需要2个用户),记录具体某位用户的四则运算历史得分(需要有序号,第几次,得分时间),需要进行持久化存储学生信息以及其对应每一次的得分情况,并且实现查询历史得分,用java序列化和反序列化的方式存储学生和得分数据
时间: 2024-03-05 11:52:29 浏览: 49
好的,我已经了解您的问题。首先,我需要确认您的问题是关于Java编程的,是吗? 如果是的话,我可以为您提供以下解决方案:
1. 创建一个User类,包含用户名和密码等信息,同时实现序列化接口,以便进行持久化存储。
```
import java.io.Serializable;
public class User implements Serializable {
private static final long serialVersionUID = 1L;
private String username;
private String password;
public User(String username, String password) {
this.username = username;
this.password = password;
}
public String getUsername() {
return username;
}
public void setUsername(String username) {
this.username = username;
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
}
```
2. 创建一个Score类,包含学生得分信息,同时实现序列化接口,以便进行持久化存储。
```
import java.io.Serializable;
import java.util.Date;
public class Score implements Serializable {
private static final long serialVersionUID = 1L;
private int id;
private String username;
private int score;
private Date time;
public Score(int id, String username, int score, Date time) {
this.id = id;
this.username = username;
this.score = score;
this.time = time;
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getUsername() {
return username;
}
public void setUsername(String username) {
this.username = username;
}
public int getScore() {
return score;
}
public void setScore(int score) {
this.score = score;
}
public Date getTime() {
return time;
}
public void setTime(Date time) {
this.time = time;
}
}
```
3. 创建一个持久化管理类,用于实现学生和得分数据的持久化存储和查询。
```
import java.io.*;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
public class PersistenceManager {
private static final String USER_FILE_PATH = "users.dat";
private static final String SCORE_FILE_PATH = "scores.dat";
public static void saveUser(List<User> userList) throws IOException {
FileOutputStream fos = new FileOutputStream(USER_FILE_PATH);
ObjectOutputStream oos = new ObjectOutputStream(fos);
oos.writeObject(userList);
oos.close();
fos.close();
}
public static List<User> loadUser() throws IOException, ClassNotFoundException {
List<User> userList = new ArrayList<User>();
FileInputStream fis = new FileInputStream(USER_FILE_PATH);
ObjectInputStream ois = new ObjectInputStream(fis);
Object obj = ois.readObject();
if (obj instanceof List) {
userList = (List<User>) obj;
}
ois.close();
fis.close();
return userList;
}
public static void saveScore(List<Score> scoreList) throws IOException {
FileOutputStream fos = new FileOutputStream(SCORE_FILE_PATH);
ObjectOutputStream oos = new ObjectOutputStream(fos);
oos.writeObject(scoreList);
oos.close();
fos.close();
}
public static List<Score> loadScore() throws IOException, ClassNotFoundException {
List<Score> scoreList = new ArrayList<Score>();
FileInputStream fis = new FileInputStream(SCORE_FILE_PATH);
ObjectInputStream ois = new ObjectInputStream(fis);
Object obj = ois.readObject();
if (obj instanceof List) {
scoreList = (List<Score>) obj;
}
ois.close();
fis.close();
return scoreList;
}
public static void addScore(String username, int score) throws IOException, ClassNotFoundException {
List<Score> scoreList = loadScore();
int id = scoreList.size() + 1;
Score newScore = new Score(id, username, score, new Date());
scoreList.add(newScore);
saveScore(scoreList);
}
public static List<Score> queryScoreByUsername(String username) throws IOException, ClassNotFoundException {
List<Score> scoreList = loadScore();
List<Score> result = new ArrayList<Score>();
for (Score score : scoreList) {
if (score.getUsername().equalsIgnoreCase(username)) {
result.add(score);
}
}
return result;
}
}
```
4. 创建一个测试类,进行数据存储和查询操作的测试。
```
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
public class Test {
public static void main(String[] args) throws IOException, ClassNotFoundException {
List<User> userList = new ArrayList<User>();
userList.add(new User("user1", "123456"));
userList.add(new User("user2", "123456"));
PersistenceManager.saveUser(userList);
List<Score> scoreList = new ArrayList<Score>();
scoreList.add(new Score(1, "user1", 80, null));
scoreList.add(new Score(2, "user1", 90, null));
scoreList.add(new Score(3, "user2", 85, null));
PersistenceManager.saveScore(scoreList);
List<User> loadedUserList = PersistenceManager.loadUser();
System.out.println(loadedUserList);
List<Score> loadedScoreList = PersistenceManager.loadScore();
System.out.println(loadedScoreList);
List<Score> queryResult = PersistenceManager.queryScoreByUsername("user1");
System.out.println(queryResult);
}
}
```
以上就是一个实现用户管理和得分记录的简单示例,您可以根据自己的需求进行扩展和优化。
阅读全文
相关推荐
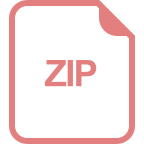




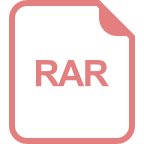

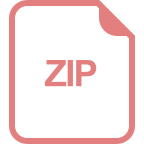
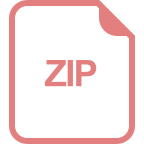
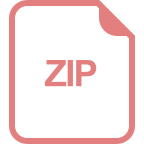
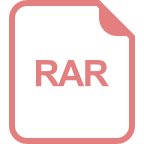
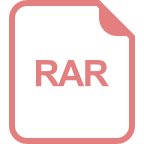
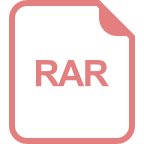
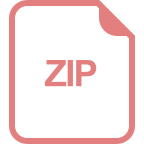
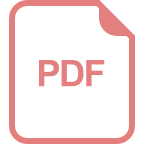