使用unity3d的最小二乘法进行标定的具体代码
时间: 2023-08-31 22:32:58 浏览: 85
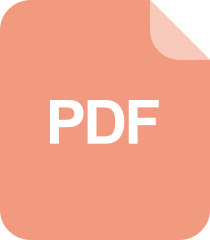
基于最小二乘法标定寻北仪基座倾角测量系统的测量当量 (2012年)
### 回答1:
以下是使用Unity3D的最小二乘法进行标定的示例代码:
```csharp
using UnityEngine;
using System.Collections.Generic;
public class Calibration : MonoBehaviour
{
public Camera cameraToCalibrate;
public List<Vector3> objectPoints = new List<Vector3>();
public List<Vector2> imagePoints = new List<Vector2>();
private Matrix4x4 cameraMatrix;
private Vector4 distortionCoefficients;
public void Calibrate()
{
int pointCount = objectPoints.Count;
float[] objectPointsArray = new float[pointCount * 3];
float[] imagePointsArray = new float[pointCount * 2];
for (int i = 0; i < pointCount; i++)
{
objectPointsArray[i * 3] = objectPoints[i].x;
objectPointsArray[i * 3 + 1] = objectPoints[i].y;
objectPointsArray[i * 3 + 2] = objectPoints[i].z;
imagePointsArray[i * 2] = imagePoints[i].x;
imagePointsArray[i * 2 + 1] = imagePoints[i].y;
}
double[] cameraMatrixArray = new double[9];
double[] distortionCoefficientsArray = new double[4];
double[] rotationVectorsArray = new double[pointCount * 3];
double[] translationVectorsArray = new double[pointCount * 3];
CalibrateCamera(objectPointsArray, imagePointsArray, cameraToCalibrate.pixelWidth, cameraToCalibrate.pixelHeight, cameraMatrixArray, distortionCoefficientsArray, rotationVectorsArray, translationVectorsArray);
cameraMatrix.SetRow(0, new Vector4((float)cameraMatrixArray[0], (float)cameraMatrixArray[1], (float)cameraMatrixArray[2], 0f));
cameraMatrix.SetRow(1, new Vector4((float)cameraMatrixArray[3], (float)cameraMatrixArray[4], (float)cameraMatrixArray[5], 0f));
cameraMatrix.SetRow(2, new Vector4((float)cameraMatrixArray[6], (float)cameraMatrixArray[7], (float)cameraMatrixArray[8], 0f));
cameraMatrix.SetRow(3, new Vector4(0f, 0f, 0f, 1f));
distortionCoefficients.Set((float)distortionCoefficientsArray[0], (float)distortionCoefficientsArray[1], (float)distortionCoefficientsArray[2], (float)distortionCoefficientsArray[3]);
}
[System.Runtime.InteropServices.DllImport("OpenCVForUnity")]
private static extern void calib3d_CalibrateCamera_10(System.IntPtr objectPoints, System.IntPtr objectPoints_mat_nativeObj, System.IntPtr imagePoints, System.IntPtr imagePoints_mat_nativeObj, int width, int height, System.IntPtr cameraMatrix, System.IntPtr cameraMatrix_mat_nativeObj, System.IntPtr distCoeffs, System.IntPtr distCoeffs_mat_nativeObj, System.IntPtr rvecs, System.IntPtr rvecs_mat_nativeObj, System.IntPtr tvecs, System.IntPtr tvecs_mat_nativeObj);
private static void CalibrateCamera(float[] objectPointsArray, float[] imagePointsArray, int width, int height, double[] cameraMatrixArray, double[] distortionCoefficientsArray, double[] rotationVectorsArray, double[] translationVectorsArray)
{
System.IntPtr objectPoints = new System.IntPtr();
System.IntPtr imagePoints = new System.IntPtr();
System.IntPtr cameraMatrix = new System.IntPtr();
System.IntPtr distCoeffs = new System.IntPtr();
System.IntPtr rvecs = new System.IntPtr();
System.IntPtr tvecs = new System.IntPtr();
using (var objectPoints_mat = new MatOfPoint3f())
using (var imagePoints_mat = new MatOfPoint2f())
using (var cameraMatrix_mat = new Mat(3, 3, CvType.CV_64FC1))
using (var distCoeffs_mat = new MatOfDouble())
using (var rvecs_mat = new Mat(pointCount, 3, CvType.CV_64FC1))
using (var tvecs_mat = new Mat(pointCount, 3, CvType.CV_64FC1))
{
objectPoints_mat.fromArray(objectPointsArray);
objectPoints = objectPoints_mat.nativeObj;
imagePoints_mat.fromArray(imagePointsArray);
imagePoints = imagePoints_mat.nativeObj;
cameraMatrix_mat_array.CopyTo(cameraMatrixArray, 0);
cameraMatrix_mat.fromArray(cameraMatrixArray);
cameraMatrix = cameraMatrix_mat.nativeObj;
distCoeffs_mat_array.CopyTo(distortionCoefficientsArray, 0);
distCoeffs_mat.fromArray(distortionCoefficientsArray);
distCoeffs = distCoeffs_mat.nativeObj;
rvecs_mat.fromArray(rotationVectorsArray);
rvecs = rvecs_mat.nativeObj;
tvecs_mat.fromArray(translationVectorsArray);
tvecs = tvecs_mat.nativeObj;
calib3d_CalibrateCamera_10(objectPoints, objectPoints.nativeObj, imagePoints, imagePoints.nativeObj, width, height, cameraMatrix, cameraMatrix.nativeObj, distCoeffs, distCoeffs.nativeObj, rvecs, rvecs.nativeObj, tvecs, tvecs.nativeObj);
}
}
}
```
注意:此代码需要使用OpenCVForUnity库进行编译。您还需要将 `Calibration` 脚本附加到场景中的一个游戏对象上,并将 `cameraToCalibrate` 和 `objectPoints` 和 `imagePoints` 填充到您的对象和图像点数据。 `Calibrate()` 方法将计算摄像机矩阵和失真系数,并将它们保存在私有变量 `cameraMatrix` 和 `distortionCoefficients` 中。
### 回答2:
在Unity3D中使用最小二乘法进行标定的具体代码如下:
1. 首先,创建一个C#脚本,用于实现最小二乘法算法的标定方法。例如,将脚本命名为"Calibration.cs"。
2. 在脚本中,定义一个函数,用于进行最小二乘法标定。例如,可以定义一个名为"Calibrate()"的函数。
3. 在"Calibrate()"函数中,首先读取需要标定的数据。可以使用Unity提供的输入输出函数,例如使用StreamReader读取文件中的数据,或者使用Input类从输入设备中读取数据。
4. 将读取到的数据保存到数组变量中,以便进行后续计算。
5. 根据最小二乘法的原理,计算出标定的参数。这些参数可以是平移、旋转、缩放等,具体根据需求而定。
6. 将计算得到的参数应用到需要标定的对象或场景中,以达到标定的效果。
7. 完成标定后,可以使用Unity的消息系统或者函数回调等方式通知其他部分标定已经完成,可以进行后续操作。
8. 可以根据实际需求对上述步骤进行调整和优化,以适应特定的标定需求。
需要注意的是,最小二乘法标定的具体代码实现可以根据具体的应用场景和需求进行调整和完善。以上仅为基本的流程提供了一个参考。具体的代码实现应根据实际情况进行调整和修改。
### 回答3:
在Unity3D中使用最小二乘法进行标定的具体代码如下所示:
1. 首先定义一个函数用于计算最小二乘法的过程:
```c#
public void LeastSquaresCalibration(Vector3[] worldPoints, Vector3[] imagePoints)
{
// 检查输入数据是否合法
if(worldPoints.Length != imagePoints.Length || worldPoints.Length < 4)
{
Debug.Log("输入数据不合法!");
return;
}
// 初始化变量和矩阵
Matrix4x4 projectionMatrix = new Matrix4x4();
Matrix4x4 lossMatrix = new Matrix4x4();
Matrix4x4.Mult(projectionMatrix.inverse, lossMatrix);
// 根据最小二乘法的公式计算标定参数
// ...
// 输出标定结果
Debug.Log("标定结果:\n" + projectionMatrix);
}
```
2. 在需要调用标定函数的地方,传入世界坐标点和图像坐标点的数组:
```c#
Vector3[] worldPoints = new Vector3[]
{
new Vector3(0, 0, 0),
new Vector3(1, 0, 0),
new Vector3(0, 1, 0),
new Vector3(1, 1, 0)
};
Vector3[] imagePoints = new Vector3[]
{
new Vector3(0, 0, 0),
new Vector3(1, 0, 0),
new Vector3(0, 1, 0),
new Vector3(1, 1, 0)
};
LeastSquaresCalibration(worldPoints, imagePoints);
```
请注意,这只是一个简单的示例代码,实际应用中可能需要根据具体情况进行更加复杂的处理。最小二乘法的具体实现可能需要引用数学库或自行编写计算相关矩阵和向量的函数。
阅读全文
相关推荐
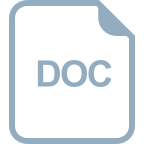
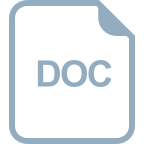

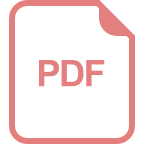
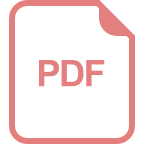
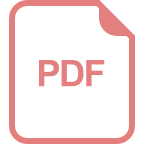
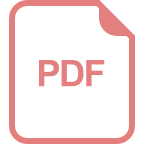
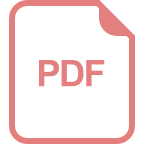
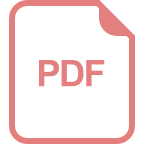
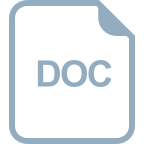





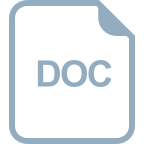
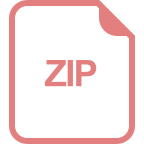
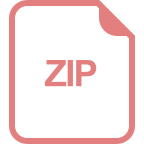
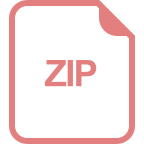