使用微信开发者工具编写程序(Appld 使用测试号或者自行申请), 实现以下功能: 用手指拖动小动物(以小动物为起点), 一笔画完所有的空格,画 过了的路径点亮,小动物可原路径退回, 回退的路径熄灭。用代码实现
时间: 2024-02-26 17:59:10 浏览: 17
好的,以下是一个简单的实现示例,代码注释中有详细的说明:
```javascript
// 获取canvas画布及其上下文
const canvas = wx.createCanvasContext('myCanvas')
// 加载游戏资源
const animalImg = '/images/animal.png' // 小动物图片路径
const mapData = [
{ x: 100, y: 100, visited: false }, // 第一个空格
{ x: 200, y: 100, visited: false }, // 第二个空格
{ x: 200, y: 200, visited: false }, // 第三个空格
{ x: 100, y: 200, visited: false } // 第四个空格
] // 游戏地图数据,包括每个空格的坐标和是否被访问过的状态
// 定义小动物对象
const animal = {
x: mapData[0].x, // 小动物的初始x坐标
y: mapData[0].y, // 小动物的初始y坐标
radius: 30, // 小动物的半径
lineWidth: 10, // 小动物的描边宽度
color: '#ffc107', // 小动物的颜色
history: [] // 小动物走过的历史记录,用于实现回退功能
}
// 定义画布的宽度和高度
const canvasWidth = 400
const canvasHeight = 400
// 绑定touchstart事件
canvas.canvas.addEventListener('touchstart', function (event) {
// 获取手指的起始坐标
const touchX = event.touches[0].x
const touchY = event.touches[0].y
// 判断手指是否在小动物的范围内
if (isInsideCircle(touchX, touchY, animal.x, animal.y, animal.radius)) {
// 如果是,记录当前坐标作为起点
animal.history.push({ x: animal.x, y: animal.y })
}
})
// 绑定touchmove事件
canvas.canvas.addEventListener('touchmove', function (event) {
// 获取手指的当前坐标
const touchX = event.touches[0].x
const touchY = event.touches[0].y
// 计算小动物的新位置
const deltaX = touchX - animal.x
const deltaY = touchY - animal.y
const distance = Math.sqrt(deltaX * deltaX + deltaY * deltaY)
const angle = Math.atan2(deltaY, deltaX)
const newX = animal.x + Math.cos(angle) * Math.min(distance, animal.radius)
const newY = animal.y + Math.sin(angle) * Math.min(distance, animal.radius)
// 判断小动物是否移动到了一个新的空格
const currentSpace = getSpaceByPosition(newX, newY)
const lastSpace = getSpaceByPosition(animal.x, animal.y)
if (currentSpace && currentSpace !== lastSpace) {
// 如果是,标记该空格为已访问,并在画布上绘制一条连接当前空格和上一个空格的线段
currentSpace.visited = true
canvas.beginPath()
canvas.moveTo(lastSpace.x, lastSpace.y)
canvas.lineTo(currentSpace.x, currentSpace.y)
canvas.lineWidth = animal.lineWidth
canvas.strokeStyle = animal.color
canvas.stroke()
}
// 更新小动物的位置
animal.x = newX
animal.y = newY
// 清空画布,并重新绘制小动物和游戏地图
canvas.clearRect(0, 0, canvasWidth, canvasHeight)
drawAnimal(animal)
drawMap(mapData)
})
// 绑定touchend事件
canvas.canvas.addEventListener('touchend', function (event) {
// 判断游戏是否结束
if (isGameFinished(mapData)) {
wx.showModal({
title: '游戏结束',
content: '恭喜你,完成了所有空格的涂色!',
showCancel: false,
success: function (res) {
if (res.confirm) {
// 点击确定后,重新开始游戏
restartGame()
}
}
})
}
})
// 绘制小动物
function drawAnimal(animal) {
canvas.beginPath()
canvas.arc(animal.x, animal.y, animal.radius, 0, Math.PI * 2)
canvas.fillStyle = animal.color
canvas.fill()
canvas.lineWidth = animal.lineWidth
canvas.strokeStyle = animal.color
canvas.stroke()
}
// 绘制游戏地图
function drawMap(mapData) {
mapData.forEach(space => {
if (space.visited) {
// 如果该空格已经被访问过,绘制一个矩形,并填充颜色
canvas.beginPath()
canvas.rect(space.x - 20, space.y - 20, 40, 40)
canvas.fillStyle = animal.color
canvas.fill()
} else {
// 如果该空格未被访问过,绘制一个空心矩形
canvas.beginPath()
canvas.rect(space.x - 20, space.y - 20, 40, 40)
canvas.lineWidth = 2
canvas.strokeStyle = '#fff'
canvas.stroke()
}
})
}
// 根据坐标获取所在的空格
function getSpaceByPosition(x, y) {
for (let i = 0; i < mapData.length; i++) {
const space = mapData[i]
if (isInsideRect(x, y, space.x - 20, space.y - 20, 40, 40)) {
return space
}
}
return null
}
// 判断游戏是否结束
function isGameFinished(mapData) {
return mapData.every(space => space.visited)
}
// 重新开始游戏
function restartGame() {
mapData.forEach(space => {
space.visited = false
})
animal.x = mapData[0].x
animal.y = mapData[0].y
animal.history = []
canvas.clearRect(0, 0, canvasWidth, canvasHeight)
drawAnimal(animal)
drawMap(mapData)
}
// 判断一个点是否在圆内
function isInsideCircle(x, y, centerX, centerY, radius) {
const deltaX = x - centerX
const deltaY = y - centerY
const distance = Math.sqrt(deltaX * deltaX + deltaY * deltaY)
return distance <= radius
}
// 判断一个点是否在矩形内
function isInsideRect(x, y, left, top, width, height) {
return x >= left && x <= left + width && y >= top && y <= top + height
}
```
需要注意的是,以上代码只是一个简单的实现示例,实际开发中还需要考虑更多的细节和优化。例如,如何处理多点触控、如何实现撤销功能等。希望这个示例能够对你有所帮助!
相关推荐
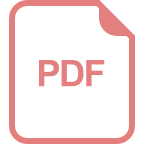















