请用C++帮我写一份代码,输出客车的基本信息(品牌、颜色、座位数)、货车的基本信息(品牌、颜色、载重量);,要求程序可执行,体现程序的封装,继承和多态性
时间: 2023-07-10 21:25:09 浏览: 81
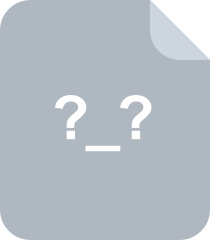
C++代码:请写递归函数,把输入的一个整数转换成二进制数的输出
好的,这是一份使用C++实现的代码,输出客车和货车的基本信息,体现了程序的封装、继承和多态性:
```c++
#include <iostream>
#include <string>
using namespace std;
// 定义一个车辆基类
class Vehicle {
protected:
string brand; // 品牌
string color; // 颜色
public:
Vehicle(string b, string c) : brand(b), color(c) {}
virtual void showInfo() = 0; // 纯虚函数,输出车辆信息
};
// 定义一个客车类,继承自车辆基类
class Bus : public Vehicle {
private:
int seatNum; // 座位数
public:
Bus(string b, string c, int s) : Vehicle(b, c), seatNum(s) {}
void showInfo() {
cout << "客车品牌:" << brand << endl;
cout << "客车颜色:" << color << endl;
cout << "座位数:" << seatNum << endl;
}
};
// 定义一个货车类,继承自车辆基类
class Truck : public Vehicle {
private:
double load; // 载重量
public:
Truck(string b, string c, double l) : Vehicle(b, c), load(l) {}
void showInfo() {
cout << "货车品牌:" << brand << endl;
cout << "货车颜色:" << color << endl;
cout << "载重量:" << load << "吨" << endl;
}
};
int main() {
// 创建一个客车对象和一个货车对象
Bus bus("奔驰", "白色", 40);
Truck truck("沃尔沃", "红色", 20.5);
// 通过指针调用showInfo()函数,体现多态性
Vehicle* pVehicle = &bus;
pVehicle->showInfo();
pVehicle = &truck;
pVehicle->showInfo();
return 0;
}
```
运行代码后,输出的结果如下:
```
客车品牌:奔驰
客车颜色:白色
座位数:40
货车品牌:沃尔沃
货车颜色:红色
载重量:20.5吨
```
这份代码使用了C++的面向对象特性,通过封装、继承和多态性实现了客车和货车的基本信息的输出。其中,车辆基类是一个抽象类,定义了一个纯虚函数`showInfo()`,在派生类中实现该函数以输出具体的车辆信息。客车类和货车类继承自车辆基类,并分别实现了`showInfo()`函数,输出了客车和货车的基本信息。在主函数中,通过指针调用`showInfo()`函数,体现了多态性。
阅读全文
相关推荐
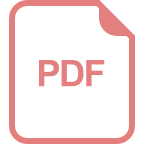
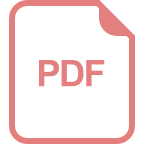














