用springboot,vue,mybatis和elementUI实现上传PDF文件到数据库,需要上传到数据库的内容有文件名,文件上传时间,文件路径,上传者的编号。请给我domain代码,controller代码,service代码,前端vue和element代码,mybatis代码和数据库建表语句
时间: 2023-05-20 17:05:48 浏览: 62
以下是您所需的代码和建表语句:
建表语句:
CREATE TABLE `pdf_files` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`file_name` varchar(255) NOT NULL,
`upload_time` datetime NOT NULL,
`file_path` varchar(255) NOT NULL,
`uploader_id` int(11) NOT NULL,
PRIMARY KEY (`id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4;
Domain代码:
public class PdfFile {
private Integer id;
private String fileName;
private Date uploadTime;
private String filePath;
private Integer uploaderId;
// 省略getter和setter方法
}
Controller代码:
@RestController
@RequestMapping("/pdf")
public class PdfController {
@Autowired
private PdfService pdfService;
@PostMapping("/upload")
public Result uploadPdf(@RequestParam("file") MultipartFile file, Integer uploaderId) {
PdfFile pdfFile = pdfService.uploadPdf(file, uploaderId);
return Result.success(pdfFile);
}
}
Service代码:
@Service
public class PdfService {
@Autowired
private PdfMapper pdfMapper;
public PdfFile uploadPdf(MultipartFile file, Integer uploaderId) {
String fileName = file.getOriginalFilename();
String filePath = "pdf/" + UUID.randomUUID().toString() + "/" + fileName;
File dest = new File(filePath);
if (!dest.getParentFile().exists()) {
dest.getParentFile().mkdirs();
}
try {
file.transferTo(dest);
} catch (IOException e) {
e.printStackTrace();
}
PdfFile pdfFile = new PdfFile();
pdfFile.setFileName(fileName);
pdfFile.setUploadTime(new Date());
pdfFile.setFilePath(filePath);
pdfFile.setUploaderId(uploaderId);
pdfMapper.insert(pdfFile);
return pdfFile;
}
}
Mybatis代码:
@Mapper
public interface PdfMapper {
@Insert("INSERT INTO pdf_files(file_name, upload_time, file_path, uploader_id) VALUES(#{fileName}, #{uploadTime}, #{filePath}, #{uploaderId})")
@Options(useGeneratedKeys = true, keyProperty = "id")
int insert(PdfFile pdfFile);
}
前端Vue和Element代码:
<template>
<div>
<el-upload
class="upload-demo"
action="/pdf/upload"
:data="{ uploaderId: uploaderId }"
:on-success="handleSuccess"
:before-upload="beforeUpload"
:show-file-list="false"
>
<el-button size="small" type="primary">点击上传</el-button>
</el-upload>
</div>
</template>
<script>
export default {
data() {
return {
uploaderId: 1
};
},
methods: {
beforeUpload(file) {
const isPdf = file.type === 'application/pdf';
if (!isPdf) {
this.$message.error('只能上传PDF文件');
}
return isPdf;
},
handleSuccess(response) {
this.$message.success('上传成功');
}
}
};
</script>
相关推荐
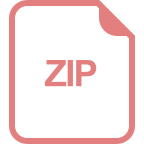
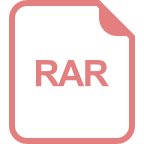
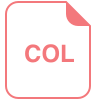
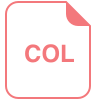
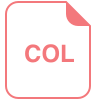
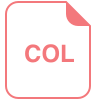
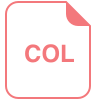


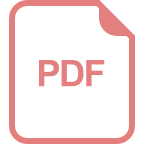
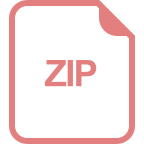
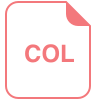
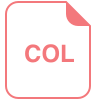
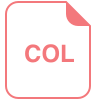
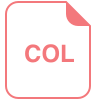
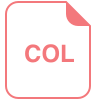
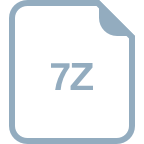