用c语言编写“链表数据存入无向图的信息文件和读取文件”
时间: 2024-03-01 22:54:07 浏览: 126
好的,我可以提供一些思路和代码示例。
首先,我们需要定义一个链表节点和图节点的结构体,它们的定义与前面提到的一样。
```c
typedef struct ListNode {
int val; // 节点值
struct ListNode *next; // 指向下一个节点的指针
} ListNode;
typedef struct GraphNode {
int val; // 节点值
struct GraphNode *next; // 指向下一个邻接节点的指针
} GraphNode;
```
然后我们可以定义一些函数来操作这个链表和图,比如创建节点、添加边等等。
```c
// 创建一个新链表节点
ListNode *new_node(int val) {
ListNode *node = (ListNode*)malloc(sizeof(ListNode));
node->val = val;
node->next = NULL;
return node;
}
// 创建一个新图节点
GraphNode *new_graph_node(int val) {
GraphNode *node = (GraphNode*)malloc(sizeof(GraphNode));
node->val = val;
node->next = NULL;
return node;
}
// 将链表转换成图
GraphNode **list_to_graph(ListNode **list, int node_count) {
GraphNode **graph = (GraphNode**)malloc(node_count * sizeof(GraphNode*));
for (int i = 0; i < node_count; i++) {
graph[i] = NULL;
}
ListNode *node = *list;
while (node) {
ListNode *cur = node;
node = node->next;
free(cur);
GraphNode *graph_node = new_graph_node(cur->val);
graph_node->next = graph[cur->val];
graph[cur->val] = graph_node;
}
*list = NULL;
return graph;
}
// 将图转换成链表
ListNode **graph_to_list(GraphNode **graph, int node_count) {
ListNode **list = (ListNode**)malloc(sizeof(ListNode*));
*list = NULL;
for (int i = 0; i < node_count; i++) {
GraphNode *node = graph[i];
while (node) {
ListNode *list_node = new_node(node->val);
list_node->next = *list;
*list = list_node;
node = node->next;
}
}
return list;
}
// 从文件中读取图的信息
GraphNode **read_graph(char *filename, int *node_count, int *edge_count) {
FILE *fp = fopen(filename, "r");
if (!fp) {
printf("Failed to open file %s\n", filename);
return NULL;
}
fscanf(fp, "%d %d", node_count, edge_count);
GraphNode **graph = (GraphNode**)malloc(*node_count * sizeof(GraphNode*));
for (int i = 0; i < *node_count; i++) {
graph[i] = NULL;
}
for (int i = 0; i < *edge_count; i++) {
int u, v;
fscanf(fp, "%d %d", &u, &v);
GraphNode *node = new_graph_node(v);
node->next = graph[u];
graph[u] = node;
node = new_graph_node(u);
node->next = graph[v];
graph[v] = node;
}
fclose(fp);
return graph;
}
// 保存图的信息到文件
void save_graph(GraphNode **graph, int node_count, int edge_count, char *filename) {
FILE *fp = fopen(filename, "w");
if (!fp) {
printf("Failed to open file %s\n", filename);
return;
}
fprintf(fp, "%d %d\n", node_count, edge_count);
for (int i = 0; i < node_count; i++) {
GraphNode *node = graph[i];
while (node) {
if (node->val > i) {
fprintf(fp, "%d %d\n", i, node->val);
}
node = node->next;
}
}
fclose(fp);
}
```
最后,我们可以在主函数中调用这些函数来创建链表、将链表转换成图、保存到文件、从文件中读取图的信息并将图转换成链表。
```c
int main() {
// 创建一个新链表
ListNode *list = NULL;
for (int i = 0; i < 5; i++) {
ListNode *node = new_node(i);
node->next = list;
list = node;
}
// 将链表转换成图
GraphNode **graph = list_to_graph(&list, 5);
// 保存到文件
save_graph(graph, 5, 4, "graph.txt");
// 从文件中读取图的信息
int node_count, edge_count;
GraphNode **new_graph = read_graph("graph.txt", &node_count, &edge_count);
// 将图转换成链表
ListNode **new_list = graph_to_list(new_graph, node_count);
// 打印链表的值
ListNode *node = *new_list;
while (node) {
printf("%d ", node->val);
node = node->next;
}
printf("\n");
return 0;
}
```
这样,我们就可以用 C 语言编写链表数据存入无向图的信息文件和读取文件了。
阅读全文
相关推荐

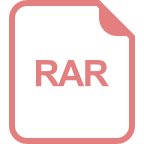
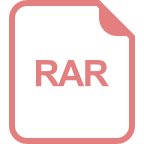
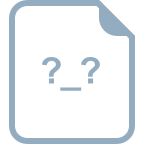
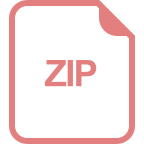
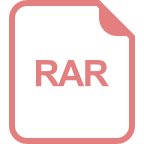
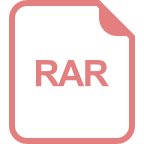