python采用遗传算法在平面内寻找到随机生成的五个点距离最近的唯一最佳选址
时间: 2024-02-19 07:59:26 浏览: 110
这个问题可以采用遗传算法来解决。遗传算法是一种优化算法,可以在搜索空间中寻找最优解。它的基本思想是通过模拟生物进化的过程,逐代筛选出对问题最优的解。
具体步骤如下:
1. 确定问题的适应度函数:在这个问题中,适应度函数可以定义为选址方案的总距离。
2. 初始化种群:生成多个随机的选址方案,并将它们存储在一个列表中。
3. 评估每个个体的适应度:计算每个选址方案的总距离,并将它们按照适应度从高到低排序。
4. 选择优秀的个体:从种群中选择若干个适应度高的个体作为“父代”,用于产生下一代的个体。
5. 交叉与变异:对父代进行交叉和变异操作,产生新的个体。
6. 评估新一代个体的适应度,并将它们按照适应度从高到低排序。
7. 重复步骤 4 到步骤 6,直到达到指定的停止条件。
8. 最终得到的种群中,适应度最高的个体即为最佳选址方案。
下面是一个示例代码,可以帮助您更好地理解:
```python
import random
import math
# 生成随机点
points = [(random.uniform(0, 100), random.uniform(0, 100)) for i in range(5)]
# 计算距离矩阵
dist_matrix = [[math.sqrt((points[i][0] - points[j][0]) ** 2 + (points[i][1] - points[j][1]) ** 2) for j in range(5)] for i in range(5)]
# 定义适应度函数
def fitness(solution):
total_distance = 0
for i in range(4):
total_distance += dist_matrix[solution[i]][solution[i+1]]
return total_distance
# 初始化种群
population = [list(range(5)) for i in range(10)]
# 设置停止条件
max_generation = 100
tolerance = 1e-6
best_fitness = float('inf')
# 进化过程
for generation in range(max_generation):
# 计算适应度
fitness_values = [fitness(solution) for solution in population]
sorted_population = [x for _, x in sorted(zip(fitness_values, population))]
sorted_fitness = sorted(fitness_values)
# 更新最佳解
if sorted_fitness[0] < best_fitness:
best_fitness = sorted_fitness[0]
best_solution = sorted_population[0]
# 判断是否达到停止条件
if generation > 0 and abs(sorted_fitness[0] - sorted_fitness[-1]) < tolerance:
break
# 选择
parents = sorted_population[:4]
# 交叉
offspring = []
for i in range(6):
parent1, parent2 = random.sample(parents, 2)
index1, index2 = sorted(random.sample(range(5), 2))
child = [-1] * 5
child[index1:index2+1] = parent1[index1:index2+1]
for j in range(5):
if parent2[j] not in child:
for k in range(5):
if child[k] == -1:
child[k] = parent2[j]
break
offspring.append(child)
# 变异
for i in range(2):
index1, index2 = sorted(random.sample(range(5), 2))
offspring[-i-1][index1], offspring[-i-1][index2] = offspring[-i-1][index2], offspring[-i-1][index1]
# 合并种群
population = parents + offspring
# 找到最佳选址
best_site = [points[i] for i in best_solution]
print("最佳选址为:", best_site)
```
这个算法的优点是可以找到全局最优解,但是它需要进行多次迭代,并且时间复杂度较高。在实际应用中,需要根据具体情况进行选择。
阅读全文
相关推荐
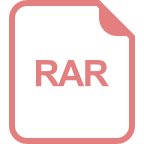
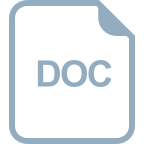
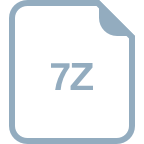
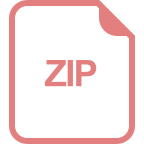
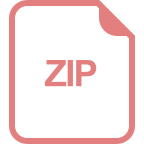
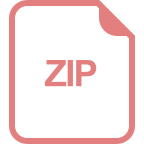
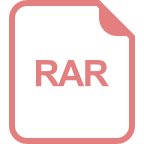
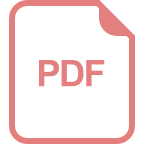
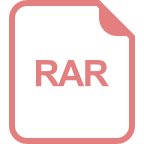
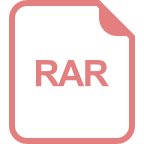
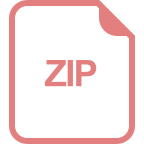
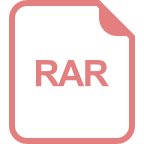
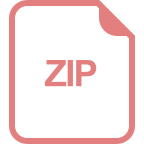